用C语言写出在单链表上按照序号进行查找的算法函数程序
时间: 2024-10-16 22:13:11 浏览: 37
在C语言中,如果想要在单链表上按序号查找元素,可以编写一个遍历链表并逐个比较每个节点序号的函数。下面是一个简单的示例,该函数接受链表的头节点和目标序号作为输入:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构
typedef struct Node {
int data;
struct Node* next;
} Node;
// 查找节点的函数
Node* findNodeByIndex(Node* head, int index) {
if (head == NULL || index < 0) {
printf("Invalid input: empty list or negative index.\n");
return NULL;
}
int count = 0; // 计数器用于追踪当前的节点序号
Node* current = head;
while (current != NULL) {
count++;
if (count == index) { // 找到了指定序号的节点
return current;
}
current = current->next;
}
// 如果遍历完整个链表都没有找到,则返回NULL
return NULL;
}
int main() {
// 假设已经有一个初始化好的链表,例如:
Node* head = createLinkedList(); // 创建链表函数略
int targetIndex = 2; // 要查找的序号
Node* result = findNodeByIndex(head, targetIndex);
if (result != NULL) {
printf("Found node at index %d with value %d.\n", targetIndex, result->data);
} else {
printf("Node not found at index %d.\n", targetIndex);
}
return 0;
}
```
在这个例子中,`createLinkedList()`函数应该提供一个初始化链表的机制,实际项目中需要根据你的需求实现。
阅读全文
相关推荐
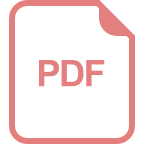
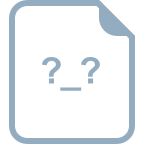
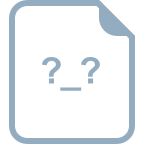















