用for循环查找 "This is a simple example string to find all occurrences of a specified character."中所有'a'
时间: 2024-10-08 09:09:59 浏览: 12
你可以使用for循环配合字符串的索引来查找给定字符串中特定字符的所有出现位置。这里是一个简单的Python示例:
```python
string = "This is a simple example string to find all occurrences of a specified character."
character_to_find = 'a'
# 初始化找到的位置列表
positions = []
for index in range(len(string)):
# 检查当前字符是否为目标字符
if string[index] == character_to_find:
# 如果是,添加到位置列表
positions.append(index)
# 打印所有找到的位置
print("字符 '{}' 出现在位置:".format(character_to_find))
for position in positions:
print(position)
```
这个代码会输出所有 'a' 字符在给定字符串中的位置。
相关问题
(Remove text) Write a program that removes all the occurrences of a specified string from a text file. For example, invoking java Exercise12_11 John filename removes the string John from the specified file. Your program should get the arguments from the command line.
Sure, I can help you with that! Here's a Java program that takes in two command line arguments - the string to be removed and the name of the file - and removes all occurrences of the specified string from the text file:
```java
import java.io.*;
public class RemoveStringFromFile {
public static void main(String[] args) {
if (args.length != 2) {
System.out.println("Usage: java RemoveStringFromFile <string-to-remove> <file-name>");
System.exit(1);
}
String strToRemove = args[0];
String fileName = args[1];
try {
BufferedReader reader = new BufferedReader(new FileReader(fileName));
StringBuffer sb = new StringBuffer();
String line;
while ((line = reader.readLine()) != null) {
line = line.replaceAll(strToRemove, "");
sb.append(line + "\n");
}
reader.close();
BufferedWriter writer = new BufferedWriter(new FileWriter(fileName));
writer.write(sb.toString());
writer.close();
System.out.println("Successfully removed all occurrences of " + strToRemove + " from " + fileName);
} catch (FileNotFoundException ex) {
System.out.println("File not found: " + fileName);
} catch (IOException ex) {
System.out.println("Error reading/writing file: " + ex.getMessage());
}
}
}
```
You can compile this program using `javac RemoveStringFromFile.java` and run it using `java RemoveStringFromFile <string-to-remove> <file-name>`. For example, if you want to remove all occurrences of the string "John" from a file called "input.txt", you can run the program like this: `java RemoveStringFromFile John input.txt`. The program will read in the contents of the file, remove all occurrences of "John", and write the modified contents back to the same file.
jupte里Find all occurrences of a substring in a given string by ignoring the case
在Jupyter Notebook中,如果你想在一个给定的字符串中查找忽略大小写的子串的所有出现,你可以使用Python内置的`re`模块(正则表达式库)。下面是一个简单的函数,它会接收一个主字符串`main_str`和一个子串`substring`,然后返回所有匹配项的列表:
```python
import re
def find_substring_occurrences(main_str, substring):
pattern = re.compile(substring, re.IGNORECASE) # 创建正则表达式模式并忽略大小写
matches = pattern.findall(main_str) # 查找所有匹配项
return matches
# 示例用法
main_str = "Hello, hello, Hello World!"
substring = "hello"
occurrences = find_substring_occurrences(main_str, substring)
print("Substring occurrences:", occurrences)
```
在这个例子中,`re.IGNORECASE`标志告诉正则表达式在搜索时不区分大小写。`pattern.findall()`会找到所有非重叠的匹配,并返回它们作为一个列表。
相关推荐
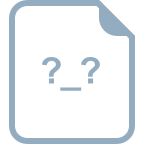
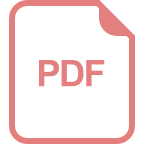
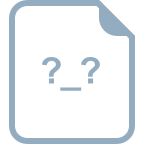





Use the method find_all() to find all hyperlinks in soup, remembering that hyperlinks are defined by the HTML tag but passed to find_all() without angle brackets; store the result in the variable a_tags. The variable a_tags is a results set: your job now is to enumerate over it, using a for loop and to print the actual URLs of the hyperlinks; to do this, for every element link in a_tags, you want to print() link.get('href').







