如何使用Python中的适当库有效地从PDF文件中提取和解析表格数据?
时间: 2024-11-23 09:40:22 浏览: 4
在Python中,可以使用一些专门用于处理PDF文档并提取其中表格数据的库,如PyPDF2、tabula-py、pandas和pdfplumber等。以下是使用这些工具的一个基本步骤:
1. **安装依赖库**:
- PyPDF2: `pip install PyPDF2` (用于读取PDF的基本结构)
- tabula-py: `pip install tabula-py` (将PDF转换为CSV或Excel格式,适合简单的表格)
- pdfplumber: `pip install pdfplumber` (更高级的库,直接解析PDF内容,包括表格)
2. **使用PyPDF2读取PDF**:
```python
import PyPDF2
with open('example.pdf', 'rb') as file:
reader = PyPDF2.PdfFileReader(file)
for page_num in range(reader.getNumPages()):
page_obj = reader.getPage(page_num)
text = page_obj.extractText()
```
3. **利用tabula-py提取表格**:
- 首先,你需要安装Java环境,因为tabula-py依赖于它。然后使用`tabula.read_pdf`函数:
```python
import tabula
df = tabula.read_pdf('example.pdf', pages='all')
```
这会返回一个Pandas DataFrame。
4. **使用pdfplumber解析表格**:
```python
from pdfplumber import PDF
with PDF('example.pdf') as pdf:
tables = pdf.pages[0].extract_tables()
for table in tables:
data = []
for row in table:
data.append([cell.strip() for cell in row])
```
这样你会得到一个二维列表,每个子列表代表一行数据。
**相关问题--:**
1. PyPDF2和pdfplumber有什么区别,在处理复杂PDF表格时应该选择哪一个?
2. 如果PDF表格有缺失的数据或复杂的布局,如何提高提取准确度?
3. tabula-py是否支持自定义页面范围或表单区域的表格提取?
阅读全文
相关推荐
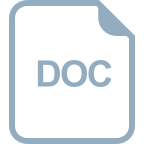
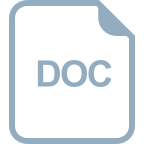
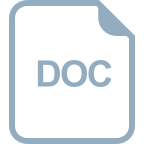
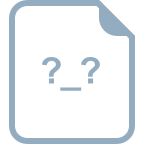
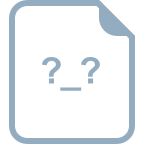
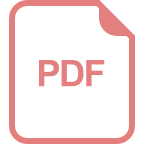
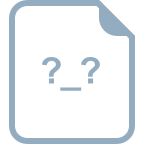
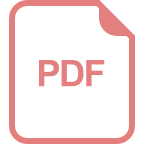
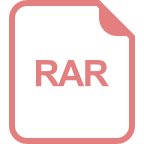









