inception pytorch
时间: 2023-11-03 22:03:29 浏览: 98
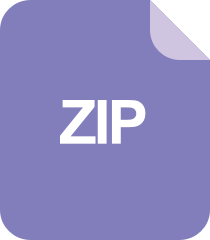
intro_to_pytorch
Inception是一种在深度学习中广泛应用的模块,最早由GoogLeNet提出并采用。它通过并行采集不同大小的卷积核来提取输入图像的特征,并将所有输出结果拼接为一个深层的特征图。这种特征提取方式丰富了特征表示,使得网络能够学到不同尺度的特征,提高了网络的性能和灵活性。
在PyTorch中,可以使用torch.nn模块来构建Inception网络。Inception网络的基本单元是一个带有1x1卷积核的Inception层,这个层由多个卷积核大小不同的子模块组成。整个网络由多个这样的Inception模块串联而成。
下面是一个使用PyTorch实现的Inception网络的示例代码:
```
class Inception(torch.nn.Module):
def __init__(self, in_channels):
super(Inception, self).__init__()
self.conv1x1 = torch.nn.Conv2d(in_channels, 10, kernel_size=1)
self.conv3x3 = torch.nn.Conv2d(in_channels, 20, kernel_size=3, padding=1)
self.conv5x5 = torch.nn.Conv2d(in_channels, 30, kernel_size=5, padding=2)
self.maxpool = torch.nn.MaxPool2d(kernel_size=3, stride=1, padding=1)
def forward(self, x):
out1 = F.relu(self.conv1x1(x))
out2 = F.relu(self.conv3x3(x))
out3 = F.relu(self.conv5x5(x))
out4 = F.relu(self.maxpool(x))
out = torch.cat((out1, out2, out3, out4), dim=1)
return out
class InceptionNet(torch.nn.Module):
def __init__(self):
super(InceptionNet, self).__init__()
self.conv1 = torch.nn.Conv2d(1, 10, kernel_size=5)
self.inception1 = Inception(in_channels=10)
self.conv2 = torch.nn.Conv2d(88, 20, kernel_size=5)
self.inception2 = Inception(in_channels=20)
self.maxpool = torch.nn.MaxPool2d(2)
self.fc = torch.nn.Linear(1408, 10)
def forward(self, x):
x = F.relu(self.maxpool(self.conv1(x)))
x = self.inception1(x)
x = F.relu(self.maxpool(self.conv2(x)))
x = self.inception2(x)
x = x.view(x.size(0), -1)
out = self.fc(x)
return out
model = InceptionNet()
```
阅读全文
相关推荐
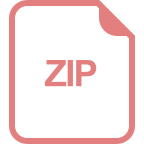


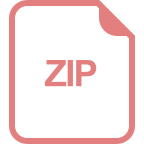
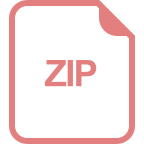
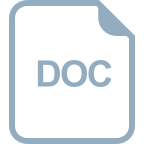









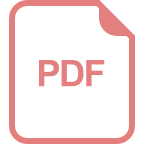
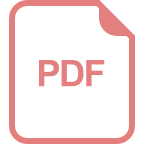