import numpy as np import matplotlib.pyplot as plt from matplotlib.colors import ListedColormap from sklearn import neighbors, datasets n_neighbors = 15 iris = datasets.load_iris() X = iris.data[:, :2] y = iris.target h = .02 # step size in the mesh cmap_light = ListedColormap(['#FFAAAA', '#AAFFAA', '#AAAAFF']) cmap_bold = ListedColormap(['#FF0000', '#00FF00', '#0000FF']) weights = 'distance' clf = neighbors.KNeighborsClassifier(n_neighbors, weights)() clf.fit(X, y) x_min, x_max = X[:, 0].min() - 1, X[:, 0].max() + 1 y_min, y_max = X[:, 1].min() - 1, X[:, 1].max() + 1 xx, yy = np.meshgrid(np.arange(x_min, x_max, h),np.arange(y_min, y_max, h)) Z = clf.predict(np.c_[xx.ravel(), yy.ravel()]) Z = Z.reshape(xx.shape) plt.figure() plt.pcolormesh(xx, yy, Z, cmap=cmap_light) plt.scatter(X[:, 0], X[:, 1], c=y, cmap=cmap_bold, edgecolor='k', s=20) plt.xlim(xx.min(), xx.max()) plt.ylim(yy.min(), yy.max()) plt.title("3-Class classification (k = %i, weights = '%s')"% (n_neighbors,weights)) plt.show()
时间: 2024-04-18 14:30:37 浏览: 173
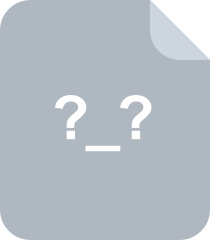
import numpy as np import matplotlib.pyplot as plt import math
这段代码是一个示例,演示了如何使用 k-最近邻算法进行三类别的分类,并使用 matplotlib 绘制分类结果的决策边界和数据点。
首先,导入必要的库,包括 numpy、matplotlib 和 sklearn。然后,设置了一些参数,如 k 值、数据集(这里使用的是鸢尾花数据集)以及步长 h。接下来,定义了两个颜色映射,用于可视化分类结果。
然后,创建了一个 KNeighborsClassifier 对象 clf,传入了 k 值和权重参数。调用 fit() 方法拟合模型,传入特征数据 X 和标签数据 y。
接着,计算了决策边界的网格点坐标,并使用 predict() 方法对网格点进行预测,得到预测结果 Z。最后,将预测结果 Z 重塑为与网格点坐标相同的形状。
最后,使用 matplotlib 绘制了分类结果的决策边界和数据点。pcolormesh() 函数用于绘制决策边界的背景颜色,scatter() 函数用于绘制数据点。设置了坐标轴范围、标题等,并调用 show() 函数显示图形。
阅读全文
相关推荐
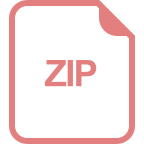
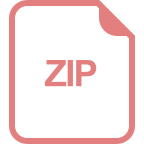













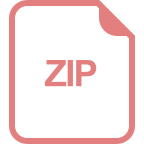
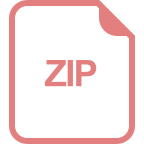
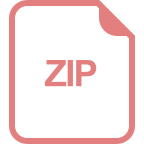