有一个目标字符串“abcde”,有一个字典数组里面存了很多字符串,从这个数组里面取字符串,拼接目标字符串,可以取一个字符串也可以取多个,拼接成功返回true,不成功返回false,用C++写
时间: 2024-12-06 17:27:13 浏览: 16
你可以使用C++的集合容器`std::set`或`std::unordered_set`来存储字典数组,并遍历查找目标字符串是否能通过拼接字典数组中的某个或某些字符串得到。这里我们假设`findSubString`是一个辅助函数,用于检查目标字符串是否可以从给定的字符串集中构造出来。下面是实现这种功能的一个示例:
```cpp
#include <iostream>
#include <vector>
#include <string>
#include <algorithm>
#include <set>
bool canConstruct(const std::string& target, const std::vector<std::string>& dictionary) {
std::set<std::string> combinedStrings; // 存储组合后的字符串
for (const auto& dict : dictionary) {
if (target.find(dict) == std::string::npos) { // 如果字典里有完整的字符串
combinedStrings.insert(dict); // 添加到集合中
} else { // 字典里的字符串是目标的一部分,尝试组合
size_t pos = 0;
while ((pos = target.find(dict, pos)) != std::string::npos) {
combinedStrings.insert(target.substr(0, pos));
pos += dict.size();
}
}
}
// 检查是否存在一种组合可以覆盖整个目标字符串
for (const auto& str : combinedStrings) {
if (str == target) {
return true;
}
}
return false;
}
// 辅助函数:检查一个字符串是否为目标字符串的子串
bool findSubString(const std::string& str, const std::string& target) {
return target.find(str) != std::string::npos;
}
int main() {
std::string target = "abcde";
std::vector<std::string> dictionary = {"a", "bc", "cd", "de"};
if (canConstruct(target, dictionary)) {
std::cout << "Target can be constructed.\n";
} else {
std::cout << "Target cannot be constructed.\n";
}
return 0;
}
```
在这个例子中,如果目标字符串"abcde"可以由字典中的"a", "bc", "cd", "de"拼接而成,函数将返回`true`;反之则返回`false`。
阅读全文
相关推荐
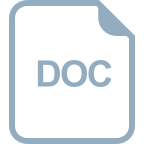
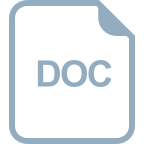
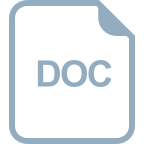
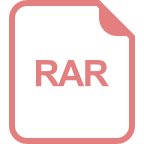
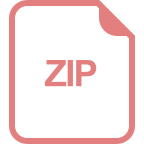
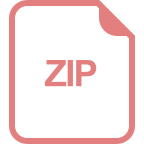
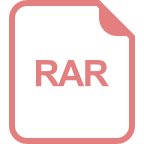
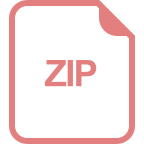
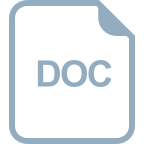
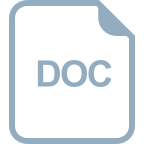
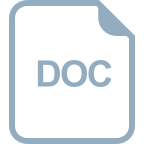
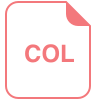





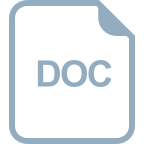
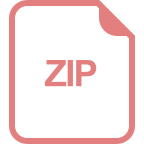