C语言 用数组完成凯撒密码的加密,待加密数组和加密后的数据保存在两个数组中,凯撒加密用函数实现,用scanf/%s接收键盘上的字符输入,用EOF结束输入
时间: 2024-11-18 07:16:15 浏览: 32
在C语言中,我们可以使用数组来实现凯撒密码(Caesar Cipher)的加密功能。凯撒密码是一种简单的替换加密技术,通过将字母表中的每个字母按照固定位移进行替换。下面是一个基本的示例,展示了如何创建一个函数,接受输入数组、偏移量以及两个存储加密结果的新数组:
```c
#include <stdio.h>
#include <string.h>
// 函数用于凯撒加密,接收输入字符串、偏移量和结果数组
void caesar_cipher(char input[], int shift, char result[], int len) {
for (int i = 0; i < len; ++i) {
if (isalpha(input[i])) { // 判断是否为字母
// 'a'到'z'的加密处理
if (input[i] >= 'a' && input[i] <= 'z') {
result[i] = ((input[i] - 'a' + shift) % 26) + 'a';
}
// 'A'到'Z'的加密处理
else if (input[i] >= 'A' && input[i] <= 'Z') {
result[i] = ((input[i] - 'A' + shift) % 26) + 'A';
}
} else {
result[i] = input[i]; // 非字母字符保持不变
}
}
}
int main() {
char plaintext[100], ciphertext[100];
int shift, length;
printf("请输入明文: ");
fgets(plaintext, sizeof(plaintext), stdin);
plaintext[strlen(plaintext)-1] = '\0'; // 去掉换行符
printf("请输入偏移量(整数): ");
scanf("%d", &shift);
length = strlen(plaintext); // 获取字符串长度
caesar_cipher(plaintext, shift, ciphertext, length);
printf("加密后的文本: ");
for (int i = 0; i < length; ++i) {
printf("%c", ciphertext[i]);
}
return 0;
}
阅读全文
相关推荐
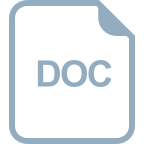
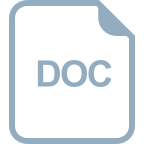
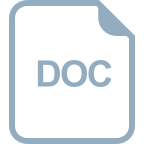
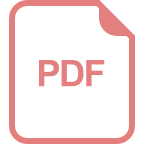
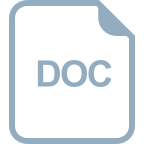
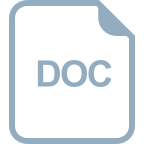
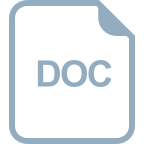
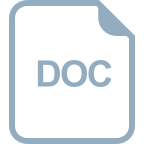
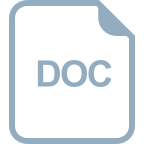









