ffmpeg SDP 解码g.711 + h264 代码示例
时间: 2023-10-11 21:09:14 浏览: 107
你可以使用FFmpeg库来进行SDP解码,并将g.711和h264进行解码。下面是一个简单的代码示例,展示了如何使用FFmpeg进行解码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <libavformat/avformat.h>
#include <libavcodec/avcodec.h>
int main(int argc, char **argv) {
AVFormatContext *fmt_ctx = NULL;
AVCodecContext *audio_dec_ctx = NULL, *video_dec_ctx = NULL;
AVCodec *audio_dec = NULL, *video_dec = NULL;
AVPacket pkt;
AVFrame *frame = NULL;
const char *sdp_filename = "input.sdp";
const char *audio_output_filename = "output.pcm";
const char *video_output_filename = "output.h264";
av_register_all();
// 打开SDP文件
if (avformat_open_input(&fmt_ctx, sdp_filename, NULL, NULL) < 0) {
fprintf(stderr, "无法打开输入文件\n");
return -1;
}
// 读取流信息
if (avformat_find_stream_info(fmt_ctx, NULL) < 0) {
fprintf(stderr, "无法获取流信息\n");
return -1;
}
// 查找音频和视频流
int audio_stream_index = -1, video_stream_index = -1;
for (int i = 0; i < fmt_ctx->nb_streams; i++) {
AVStream *stream = fmt_ctx->streams[i];
AVCodecParameters *codecpar = stream->codecpar;
if (codecpar->codec_type == AVMEDIA_TYPE_AUDIO && audio_stream_index < 0) {
audio_stream_index = i;
audio_dec = avcodec_find_decoder(codecpar->codec_id);
audio_dec_ctx = avcodec_alloc_context3(audio_dec);
avcodec_parameters_to_context(audio_dec_ctx, codecpar);
avcodec_open2(audio_dec_ctx, audio_dec, NULL);
} else if (codecpar->codec_type == AVMEDIA_TYPE_VIDEO && video_stream_index < 0) {
video_stream_index = i;
video_dec = avcodec_find_decoder(codecpar->codec_id);
video_dec_ctx = avcodec_alloc_context3(video_dec);
avcodec_parameters_to_context(video_dec_ctx, codecpar);
avcodec_open2(video_dec_ctx, video_dec, NULL);
}
if (audio_stream_index >= 0 && video_stream_index >= 0) {
break;
}
}
// 打开音频和视频输出文件
FILE *audio_output_file = fopen(audio_output_filename, "wb");
if (!audio_output_file) {
fprintf(stderr, "无法打开音频输出文件\n");
return -1;
}
FILE *video_output_file = fopen(video_output_filename, "wb");
if (!video_output_file) {
fprintf(stderr, "无法打开视频输出文件\n");
return -1;
}
// 初始化帧
frame = av_frame_alloc();
if (!frame) {
fprintf(stderr, "无法分配帧内存\n");
return -1;
}
// 解码音频和视频数据
while (av_read_frame(fmt_ctx, &pkt) >= 0) {
AVStream *stream = fmt_ctx->streams[pkt.stream_index];
AVCodecContext *dec_ctx;
if (pkt.stream_index == audio_stream_index) {
dec_ctx = audio_dec_ctx;
fwrite(pkt.data, 1, pkt.size, audio_output_file);
} else if (pkt.stream_index == video_stream_index) {
dec_ctx = video_dec_ctx;
fwrite(pkt.data, 1, pkt.size, video_output_file);
} else {
av_packet_unref(&pkt);
continue;
}
// 解码数据包
int ret = avcodec_send_packet(dec_ctx, &pkt);
if (ret < 0) {
fprintf(stderr, "错误解码数据包\n");
break;
}
while (ret >= 0) {
ret = avcodec_receive_frame(dec_ctx, frame);
if (ret == AVERROR(EAGAIN) || ret == AVERROR_EOF) {
break;
} else if (ret < 0) {
fprintf(stderr, "错误解码帧\n");
break;
}
// 处理解码后的帧
av_frame_unref(frame);
}
av_packet_unref(&pkt);
}
// 清理资源
av_frame_free(&frame);
avcodec_free_context(&audio_dec_ctx);
avcodec_free_context(&video_dec_ctx); avformat_close_input(&fmt_ctx);
fclose(audio_output_file);
fclose(video_output_file);
return 0;
}
```
你可以将上述代码保存为一个C文件,并使用FFmpeg的开发库进行编译链接。请确保你安装了FFmpeg库,并且在编译时将其链接到你的项目中。
这只是一个简单的示例,你可能需要根据你的具体需求进行修改和优化。请注意,对于音频解码后的g.711数据,你可能需要使用适当的工具将其转换为可播放的音频格式。
希望这能帮到你!如有其他问题,请随时提问。
阅读全文
相关推荐
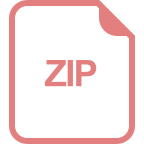
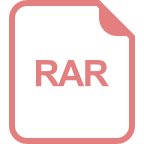
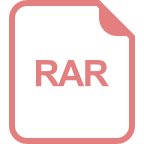
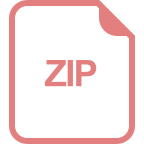
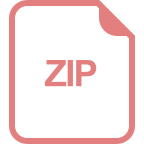
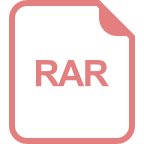
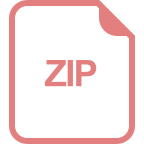
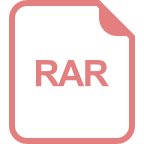
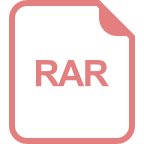
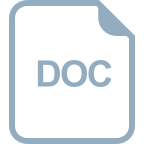
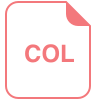
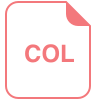



