pytest断言url
时间: 2023-12-16 11:27:43 浏览: 32
pytest可以使用assert语句进行断言,可以通过requests库获取url的响应内容,然后使用assert语句进行判断。以下是一个例子:
```python
import requests
def test_url():
url = "http://www.baidu.com"
res = requests.get(url)
assert res.status_code == 200, "请求失败"
```
在这个例子中,我们使用requests库获取了百度首页的响应内容,并使用assert语句判断响应状态码是否为200。如果状态码不为200,则会输出"请求失败"的错误信息。
相关问题
pytest接口自动化断言封装
在pytest接口自动化中,我们可以通过封装断言函数来实现断言的重用,提高代码的可维护性和可读性。下面是一个示例:
```python
import json
def assert_code(response, expected_code):
"""
封装断言状态码的函数
:param response: 接口响应
:param expected_code: 预期状态码
"""
assert response.status_code == expected_code, f"响应状态码错误,预期为{expected_code},实际为{response.status_code}"
def assert_json(response, expected_json):
"""
封装断言json响应的函数
:param response: 接口响应
:param expected_json: 预期json响应
"""
assert response.headers['Content-Type'] == 'application/json', "响应头Content-Type不是application/json"
assert response.json() == json.loads(expected_json), f"响应json内容错误,预期为{expected_json},实际为{response.text}"
```
在上面的代码中,我们封装了两个断言函数,一个用于断言状态码,一个用于断言json响应。这样,在编写测试用例时,我们只需要调用这些函数即可,如下所示:
```python
def test_login():
# 发送登录请求
response = requests.post(url, data=data)
# 断言状态码
assert_code(response, 200)
# 断言json响应
assert_json(response, '{"code": 0, "msg": "登录成功"}')
```
这样,我们就可以通过封装断言函数来实现断言的重用,提高代码的可维护性和可读性。
pytest+request
Pytest是一个Python的测试框架,它提供了简单且易于使用的方式来编写测试用例。在test中,我们可以使用内置的request对象来访问测试用例的请求信息。
通过使用request对象,我们可以获取测试用例的请求方法、URL、请求参数、请求头等信息,以便在测试中进行断言或者其他操作。
下面是一个示例,展示了如何在Pytest中使用request对象:
```python
import pytest
@pytest.fixture
def api_request(request):
# 获取请求方法
method = request.node.get_closest_marker('method').args[0]
# 获取请求URL
url = request.node.get_closest_marker('url').args[0]
# 获取请求参数
params = request.node.get_closest_marker('params').args[0]
# 获取请求头
headers = request.node.get_closest_marker('headers').args[0]
# 在这里可以进行断言或其他操作
yield
# 使用自定义标记来指定请求信息
@pytest.mark.method('GET')
@pytest.mark.url('https://api.example.com/users')
@pytest.mark.params({'page': 1, 'limit': 10})
@pytest.mark.headers({'Authorization': 'Bearer xxx'})
def test_api_request(api_request):
# 这里进行实际的测试操作
assert True
```
在上面的示例中,我们定义了一个`api_request`的fixture,它通过`request`对象获取了测试用例的请求方法、URL、请求参数和请求头。然后我们可以在`yield`之前进行断言或其他操作。接下来,我们使用自定义的标记来指定测试用例的请求信息,然后将`api_request`作为参数传入测试用例函数中。
这样,我们就可以在Pytest中使用request对象来访问测试用例的请求信息,并进行相应的操作。希望对你有所帮助!如有更多问题,请继续提问。
相关推荐
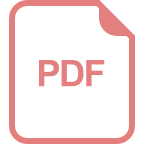
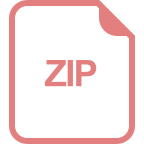
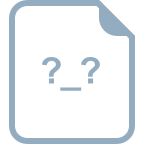












