c语言实现RSA签名的代码
时间: 2024-06-24 07:01:09 浏览: 150
在C语言中实现RSA签名通常涉及到两个主要步骤:生成密钥对和使用私钥进行签名。以下是一个简化的示例,展示了如何使用 OpenSSL 库来完成这个过程。请注意,这只是一个基础的概述,实际应用中你需要处理更多的错误检查和安全措施。
```c
#include <openssl/bio.h>
#include <openssl/rsa.h>
#include <openssl/pem.h>
#include <openssl/evp.h>
// 假设你已经有了公钥和私钥
RSA *private_key;
BIO *bioPrivateKey, *bioPublicKey;
// 函数来加载PEM格式的私钥
void load_private_key(const char *file_path) {
bioPrivateKey = BIO_new_file(file_path, "rb");
if (!bioPrivateKey) {
// 处理错误
perror("Error opening private key file");
return;
}
RSA *key = PEM_read_bio_RSAPrivateKey(bioPrivateKey, NULL, NULL, NULL);
if (!key) {
// 处理错误
BIO_free_all(bioPrivateKey);
perror("Error reading private key");
return;
}
private_key = key;
}
// 简化版的RSA签名函数,假设data是要签名的数据
int rsa_sign(char *data, int data_len) {
unsigned char digest[EVP_MAX_MD_SIZE];
unsigned int digest_len;
BIO_md_init();
BIO_md_update(bioPrivateKey, data, data_len);
BIO_md_final(digest, &digest_len);
unsigned char signature[RSA_size(private_key)];
int sig_len = RSA_sign(NID_sha256, digest, digest_len, signature, NULL);
if (sig_len <= 0) {
// 处理错误
BIO_free_all(bioPrivateKey);
return -1;
}
// 签名已计算,你可以将其存储或发送
// ...
BIO_free_all(bioPrivateKey);
return 0;
}
// 示例用法
int main() {
load_private_key("private_key.pem");
char *data_to_sign = "Hello, RSA!";
int data_len = strlen(data_to_sign);
if (rsa_sign(data_to_sign, data_len) == 0) {
printf("Signature successful.\n");
} else {
printf("Signature failed.\n");
}
return 0;
}
```
阅读全文
相关推荐
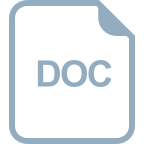
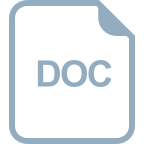
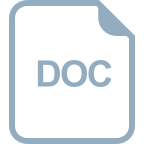

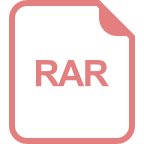
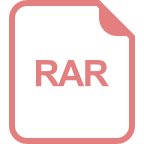
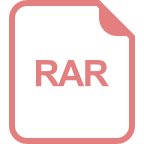
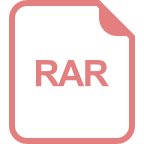
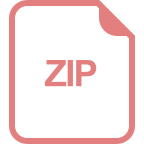
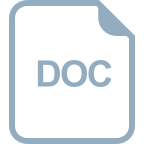
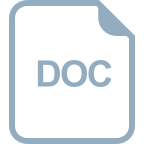
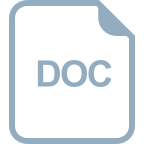
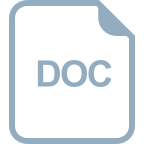


