写一个求两个有序链表序列的交集的c语言代码
时间: 2024-10-14 19:11:13 浏览: 11
在C语言中,可以使用双指针法来解决这个问题。首先,我们将两个链表的头节点分别指向两个有序链表,并将它们的指针设置为第一个元素。然后我们创建一个新的链表来存储交集。下面是算法步骤:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
typedef struct ListNode {
int val;
struct ListNode *next;
} ListNode;
// 创建新节点函数
ListNode* createNode(int data) {
ListNode *node = (ListNode*)malloc(sizeof(ListNode));
node->val = data;
node->next = NULL;
return node;
}
// 求两个链表交集
ListNode* intersectTwoLists(ListNode* list1, ListNode* list2) {
ListNode *slow1 = list1, *fast1 = list1, *slow2 = list2, *fast2 = list2;
// 如果list1或list2为空,则直接返回另一个链表的头节点
if (!list1 || !list2) {
return list1 ? list2 : list1;
}
while (fast1 && fast2) {
if (fast1->val > fast2->val) {
slow1 = slow1->next; // 向前移动较短链表的慢指针
if (!slow1) break; // 如果短链表遍历完,说明无交集
} else if (fast1->val < fast2->val) {
slow2 = slow2->next; // 同样向前移动较长链表的慢指针
if (!slow2) break; // 若长链表遍历完,说明无交集
} else { // 否则,找到相同值,添加到结果链表并同时向后移动指针
ListNode *result = createNode(fast1->val);
slow1 = slow1->next;
fast1 = fast1->next;
result->next = intersectTwoLists(slow1, fast2);
return result;
}
}
// 返回交集链表,注意这里也可能有一个链表未遍历完
return slow1 ? intersectTwoLists(NULL, slow1) : intersectTwoLists(slow2, NULL);
}
// 打印链表
void printList(ListNode* head) {
while (head) {
printf("%d ", head->val);
head = head->next;
}
printf("\n");
}
int main() {
// 测试示例,自行替换实际链表数据
ListNode *list1 = createNode(4); list1->next = createNode(5); list1->next->next = createNode(6); // [4, 5, 6]
ListNode *list2 = createNode(1); list2->next = createNode(3); list2->next->next = createNode(5); // [1, 3, 5]
ListNode *intersection = intersectTwoLists(list1, list2);
printList(intersection);
return 0;
}
```
这个程序会找出两个有序链表中的公共元素,并将它们连接成一个新的链表。运行`main()`函数后,它会打印出交集链表[5]。
阅读全文
相关推荐
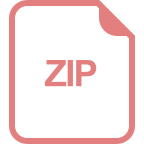
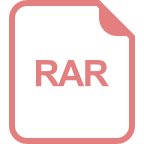
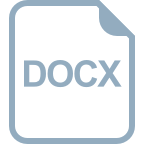




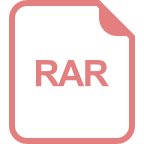
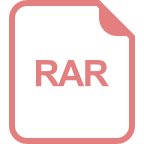
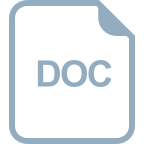
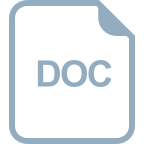
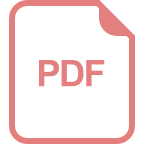
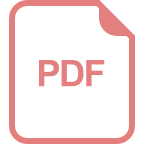
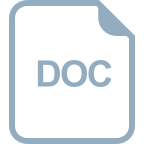
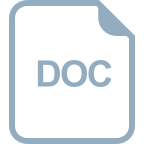
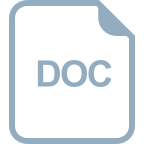
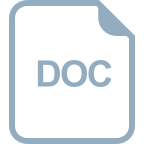
