# 构建一个迷宫通路,要求:通过栈和队列生成的是两种不同的道路。给出代码
时间: 2024-10-08 18:04:09 浏览: 32
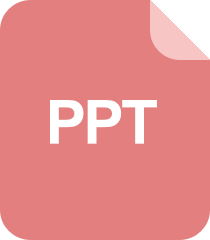
求迷宫路径算法的基本思想是-栈与队列。
在构建一个迷宫通路的过程中,通常会利用深度优先搜索(DFS)或广度优先搜索(BFS)来探索路径,而栈和队列分别代表了这两种算法的基本数据结构。这里以Python为例,展示如何分别用栈和队列生成两种不同的迷宫通路:
**使用栈(模拟DFS):**
```python
def dfs_maze(maze, start, end, path=[]):
path = path + [start]
if start == end:
return path
if start not in maze or maze[start] == 0:
return None
maze[start] = 0
for neighbor in [(i, j) for i in [-1, 0, 1] for j in [-1, 0, 1] if (i != 0 or j != 0) and (0 <= i + start[0] < len(maze)) and (0 <= j + start[1] < len(maze[0]))]:
new_path = dfs_maze(maze, neighbor, end, path)
if new_path is not None:
return new_path
return None
# 初始化迷宫矩阵(1为墙,0为空地)
maze = [[1, 1, 1], [0, 0, 1], [1, 0, 0]]
start = (0, 0)
end = (len(maze) - 1, len(maze[0]) - 1)
dfs_path = dfs_maze(maze, start, end)
```
**使用队列(模拟BFS):**
```python
from collections import deque
def bfs_maze(maze, start, end):
queue = deque([(start, [])])
while queue:
cell, path = queue.popleft()
if cell == end:
return path
if maze[cell[0]][cell[1]] == 0:
maze[cell[0]][cell[1]] = 0
queue.extend(((neighbor, path + [cell]) for neighbor in [(cell[0]+dx, cell[1]+dy) for dx, dy in [(0, 1), (1, 0), (0, -1), (-1, 0)] if 0 <= neighbor[0] < len(maze) and 0 <= neighbor[1] < len(maze[0])]))
return None
bfs_path = bfs_maze(maze, start, end)
```
在这两个例子中,`dfs_path`将表示由栈生成的一条路径,而`bfs_path`则是队列生成的另一条路径。
阅读全文
相关推荐
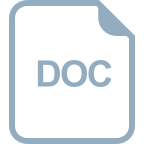
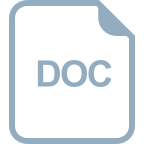


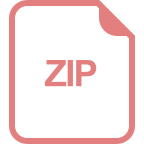
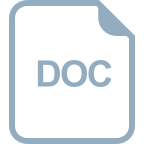
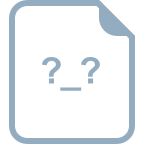
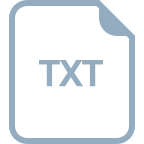
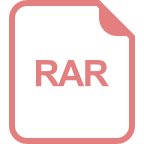
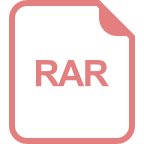
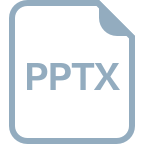
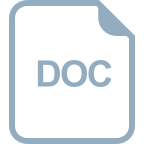
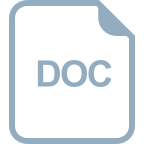
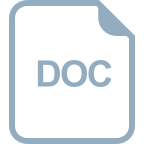
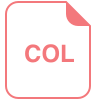
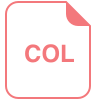
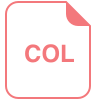