我正在编辑【python】代码,遇到了 【NameError:name'file_train'is not defined】 ,请帮我检查并改正错误点。我的原始代码如下:
时间: 2024-10-17 18:03:32 浏览: 36
这个错误提示 `NameError: name 'file_train' is not defined` 表示Python解释器找不到名为`file_train`的变量或函数。这说明你在代码中尝试引用了一个未被声明或导入的变量。以下是可能的原因及解决步骤:
1. 检查拼写:确保你引用的变量名(这里是`file_train`)书写无误,并且在整个代码文件中一致。
```python
# 检查是否有这样的定义
with open('file_train.csv', 'r') as file_train:
# 如果没有,这里应该有一个类似上面的定义
```
2. 确保变量是在适当的作用域内定义的:如果你在一个函数或循环内部试图访问`file_train`,它可能只在这个范围内可见,需要将其放在函数外部或者作为返回值传递。
3. 导入相关模块:如果`file_train`是一个模块或包里的对象,你需要先导入那个模块。
```python
import my_module # 假设my_module包含file_train
with my_module.file_train('data.txt'):
# 这里可以访问file_train
```
4. 可能是你忘记运行导入语句或读取文件的部分了,确认这部分已经被正确执行。
检查上述几点后,看看是否能解决问题。如果问题依然存在,请提供更多代码上下文以便我能提供更精确的帮助。
相关问题
def train_model(model, x_train, y_train, x_test): # 初始化model列表 model_list = [] # ... 其他代码 ... # 添加决策树模型 model_list.append(("DecisionTree", classifier)) classifier = tree.DecisionTreeClassifier() train_model(classifier, x_train, y_train, x_test) Traceback (most recent call last): File "C:\Users\lenovo\AppData\Local\Temp\ipykernel_4900\2433188095.py", line 6, in <module> model_list.append(("DecisionTree", classifier)) NameError: name 'model_list' is not defined
这个错误提示表明你在向`model_list`列表中添加模型时,`model_list`变量未被定义。在函数中,你需要在使用`model_list`变量之前先将其定义为一个空列表,例如:
```python
def train_model(model, x_train, y_train, x_test):
# 初始化model列表
model_list = []
# ... 其他代码 ...
# 添加决策树模型
model_list.append(("DecisionTree", model))
# ... 其他代码 ...
```
在这个示例中,我们将`model_list`定义为一个空列表,并使用`append()`方法将模型添加到列表中。
然后,在调用`train_model()`函数时,你需要传递正确的参数。具体而言,你需要将`classifier`对象作为第一个参数传递给函数,例如:
```python
classifier = tree.DecisionTreeClassifier()
train_model(classifier, x_train, y_train, x_test)
```
这样,你就可以正确地调用`train_model()`函数并训练分类器模型了。
代码错误:Traceback (most recent call last): File "C:\Users\ye\PycharmProjects\pythonProject\main.py", line 60, in <module> train_datagen = ImageDataGenerator(rescale=1./255, shear_range=0.2, zoom_range=0.2, horizontal_flip=True) NameError: name 'ImageDataGenerator' is not defined请写出修改后的完整代码
这个错误提示说明您的代码中没有导入`ImageDataGenerator`类,需要先从`tensorflow.keras.preprocessing.image`模块中导入该类才能使用。以下是修改后的代码:
```python
import tensorflow as tf
from tensorflow.keras.preprocessing.image import ImageDataGenerator
# 加载数据集
train_datagen = ImageDataGenerator(rescale=1./255, shear_range=0.2, zoom_range=0.2, horizontal_flip=True)
train_generator = train_datagen.flow_from_directory('dataset/train', target_size=(224, 224), batch_size=32, class_mode='binary')
test_datagen = ImageDataGenerator(rescale=1./255)
test_generator = test_datagen.flow_from_directory('dataset/test', target_size=(224, 224), batch_size=32, class_mode='binary')
# 定义模型
model = tf.keras.models.Sequential([
tf.keras.layers.Conv2D(32, (3, 3), activation='relu', input_shape=(224, 224, 3)),
tf.keras.layers.MaxPooling2D((2, 2)),
tf.keras.layers.Conv2D(64, (3, 3), activation='relu'),
tf.keras.layers.MaxPooling2D((2, 2)),
tf.keras.layers.Conv2D(128, (3, 3), activation='relu'),
tf.keras.layers.MaxPooling2D((2, 2)),
tf.keras.layers.Flatten(),
tf.keras.layers.Dense(256, activation='relu'),
tf.keras.layers.Dropout(0.5),
tf.keras.layers.Dense(1, activation='sigmoid')
])
# 编译模型
model.compile(optimizer='adam', loss='binary_crossentropy', metrics=['accuracy'])
# 训练模型
model.fit(train_generator, steps_per_epoch=len(train_generator), epochs=50, validation_data=test_generator, validation_steps=len(test_generator))
```
在这个代码中,我们从`tensorflow.keras.preprocessing.image`模块中导入了`ImageDataGenerator`类,并使用它来加载数据集。同时,我们还修改了模型的定义方式,使用了`tf.keras.models.Sequential`类来构建模型。最后,我们使用`model.fit()`方法进行模型训练。
阅读全文
相关推荐
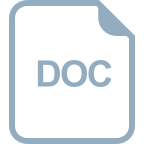
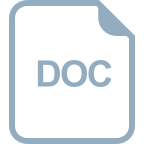
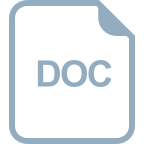
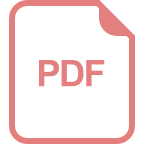
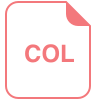
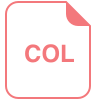
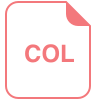
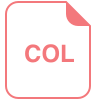
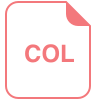
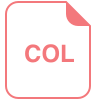
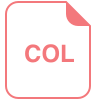
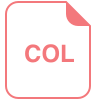





