(2)定义一个类,该类具有x和y两个静态变量,定义构造方法初始化这两个变量。再定义4个方法,分别求这两个数的和、差、乘、除结果并输出。在main()方法中,用户应能输入这两个数。
时间: 2024-10-27 20:11:47 浏览: 19
为了创建这样一个类,我们可以命名为`NumberOperations`,它包含两个静态变量`x`和`y`以及相关的计算方法。以下是这个类的设计:
```java
public class NumberOperations {
// 定义静态变量 x 和 y
public static int x;
public static int y;
// 构造方法用于初始化静态变量
public NumberOperations(int num1, int num2) {
x = num1;
y = num2;
}
// 方法求和
public static void add() {
System.out.println("The sum of x and y is: " + (x + y));
}
// 方法求差
public static void subtract() {
System.out.println("The difference between x and y is: " + (x - y));
}
// 方法求积
public static void multiply() {
System.out.println("The product of x and y is: " + (x * y));
}
// 方法求商(假设除数不为零)
public static void divide() {
if (y != 0) {
System.out.println("The quotient of x by y is: " + (x / y));
} else {
System.out.println("Cannot divide by zero.");
}
}
// 主方法,获取用户输入并调用相应方法
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter the first number: ");
int num1 = scanner.nextInt();
System.out.print("Enter the second number: ");
int num2 = scanner.nextInt();
NumberOperations obj = new NumberOperations(num1, num2);
add();
subtract();
multiply();
divide(); // 注意处理除数为零的情况
scanner.close();
}
}
```
在这个例子中,用户在运行`main()`方法时会看到提示输入两个数字,然后程序会执行相应的加、减、乘、除运算并输出结果。如果用户尝试除以零,程序将捕获异常并显示适当的错误消息。
阅读全文
相关推荐
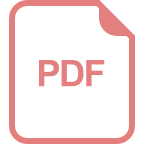
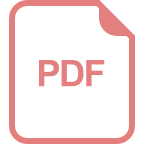
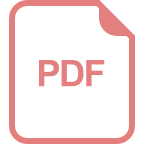















