first集合follow集合代码
时间: 2024-11-23 07:38:45 浏览: 10
在编程中,"first"和"follow"通常是指上下文无关文法(Context-Free Grammar, CFG)中的术语,它们在构造解析算法如LL解析、LR解析等中扮演重要角色。
1. **First集合**:First集合表示从某非终结符开始,可以产生的第一个标记集。例如,如果有一个非终结符A,它的First(A)包含了所有可以从A开始的最左推导出的第一个字符或标记。例如,如果A可以生成AB,AC,则First(A)可能包括B、C和ε(空字符)。
```python
# Python示例
nonterminals = {'A': ['B', 'C']}
first_sets = {}
first_sets['A'] = {token for grammar in nonterminals.values() for token in grammar} | {'ε'}
```
2. **Follow集合**:Follow集合指定了在特定位置,后续能跟哪些终结符。对于某个非终结符,其Follow集合包含了在该非终结符之后可以合法出现的所有终结符,用于确定何时关闭预测分析表。例如,在处理A -> aBc的规则时,Follow('A')可能包含{'}',因为在这个点上,我们期望找到一个结束的标记。
```python
# 示例
follow_sets = {'A': set(), 'B': {'ε'}, 'C': {'ε'}}
# 当A后面需要跟的是终结符,Follow(A)可能更新为Follow(A) | {'}'}
```
相关问题
求first集合follow集合C语言代码
在C语言中,如果我们要操作两个集合(这里假设我们简单地将它们看作是数组),"first"集合和"follow"集合通常是指某种状态机分析中的术语,例如在词法分析或者正则表达式匹配中。然而,C语言本身并不直接支持集合的数据结构,但我们可以用数组或动态数组来模拟。
以下是一个简单的例子,我们创建两个整数数组来代表两个集合:
```c
#include <stdio.h>
#define SIZE 10 // 集合大小
// 假设first集合存储了某些开始字符
int first[SIZE] = { 'a', 'b', '*' }; // 这里只是一个示例
// follow集合存储了某个字符之后可以接哪些字符
int follow[SIZE] = { 'a', 'b', 'c', 'd' }; // 类似的示例
void printCollections() {
printf("First collection (start characters): ");
for(int i = 0; i < SIZE; ++i) {
if(first[i] != '*') { // 如果不是通配符
printf("%c ", first[i]);
} else {
printf("<any character>"); // 用通配符表示任意字符
}
}
printf("\n");
printf("Follow collection (characters after a given character): ");
for(int i = 0; i < SIZE; ++i) {
if(follow[i] != '\0') { // 结束条件是遇到空字符
printf("%c ", follow[i]);
} else {
printf("<end of input>\n"); // 表示输入结束
}
}
}
int main() {
printCollections();
return 0;
}
求first和follow集合 代码
首先,需要明确一下什么是first集合和follow集合。
- first集合:对于一个文法符号,它的first集合是由它所能推导出的所有字符串的首字符组成的集合。
- follow集合:对于一个非终结符号,它的follow集合是由它所在产生式右侧的所有符号所能推导出的所有字符串的下一个字符组成的集合。
下面是求解first和follow集合的代码示例:
```python
# 定义文法符号和产生式
grammar = {
'S': ['AB', 'BC'],
'A': ['a', 'aA'],
'B': ['b', 'bB'],
'C': ['c', 'cC', '']
}
# 计算first集合
def calc_first(grammar):
first = {}
for symbol in grammar:
first[symbol] = set()
while True:
updated = False
for symbol in grammar:
for production in grammar[symbol]:
if production == '':
if '' not in first[symbol]:
first[symbol].add('')
updated = True
elif production[0] not in grammar:
if production[0] not in first[symbol]:
first[symbol].add(production[0])
updated = True
else:
for s in production:
if '' not in first[s]:
break
if s != symbol:
first[symbol] |= first[s]
if '' not in first[s]:
break
else:
if '' not in first[symbol]:
first[symbol].add('')
updated = True
if not updated:
break
return first
# 计算follow集合
def calc_follow(grammar, first):
follow = {}
for symbol in grammar:
follow[symbol] = set()
follow['S'].add('$')
while True:
updated = False
for symbol in grammar:
for production in grammar[symbol]:
for i, s in enumerate(production):
if s in grammar:
if i == len(production) - 1:
if '' not in follow[s]:
follow[s] |= follow[symbol]
updated = True
else:
for j in range(i + 1, len(production)):
if production[j] not in grammar:
if production[j] not in follow[s]:
follow[s].add(production[j])
updated = True
break
else:
follow[s] |= first[production[j]]
if '' not in first[production[j]]:
break
else:
if '' not in follow[s]:
follow[s] |= follow[symbol]
updated = True
if not updated:
break
return follow
# 测试代码
first = calc_first(grammar)
follow = calc_follow(grammar, first)
print('first集合:', first)
print('follow集合:', follow)
```
以上代码中,我们首先定义了一个文法符号和产生式的字典,然后分别计算了它们的first集合和follow集合。在计算first集合时,我们使用了一个while循环,不断更新每个符号的first集合,直到没有符号的first集合发生变化为止。在计算follow集合时,我们同样使用了一个while循环,不断更新每个符号的follow集合,直到没有符号的follow集合发生变化为止。
最后,我们将计算得到的first集合和follow集合打印出来,供测试和调试使用。
阅读全文
相关推荐
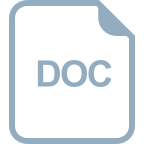
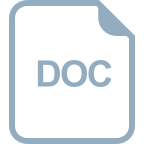
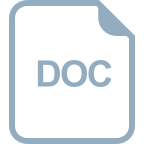
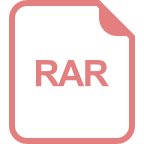
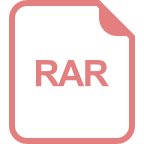
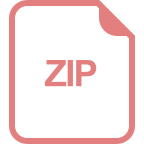








