c++map怎么重载排序规则
时间: 2024-11-15 09:14:18 浏览: 1
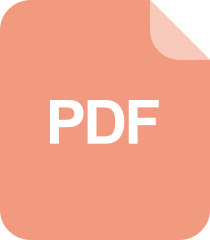
基于C++ map中key使用指针问题的详解
在C++中,`std::map` 默认使用 `std::less` 作为排序规则,这意味着它会根据键值进行升序排序。如果你想自定义排序规则,可以通过提供一个自定义的比较函数或函数对象来重载排序规则。
以下是如何重载 `std::map` 的排序规则的步骤:
1. **定义一个比较函数或函数对象**:你需要定义一个可以比较两个键值的函数或函数对象。这个函数应该返回一个布尔值,表示第一个参数是否应该排在第二个参数之前。
2. **在创建 `std::map` 时指定比较函数**:在创建 `std::map` 实例时,将你的比较函数或函数对象传递给 `std::map` 的构造函数。
### 示例代码
#### 使用函数对象
```cpp
#include <iostream>
#include <map>
// 自定义比较函数对象
struct CustomCompare {
bool operator()(const int& lhs, const int& rhs) const {
return lhs > rhs; // 降序排序
}
};
int main() {
// 使用自定义比较函数对象创建 map
std::map<int, std::string, CustomCompare> myMap;
myMap[3] = "three";
myMap[1] = "one";
myMap[2] = "two";
for (const auto& pair : myMap) {
std::cout << pair.first << " => " << pair.second << std::endl;
}
return 0;
}
```
#### 使用 Lambda 表达式(C++11及以上)
```cpp
#include <iostream>
#include <map>
int main() {
// 使用 lambda 表达式创建 map
auto comp = [](const int& lhs, const int& rhs) { return lhs > rhs; };
std::map<int, std::string, decltype(comp)> myMap(comp);
myMap[3] = "three";
myMap[1] = "one";
myMap[2] = "two";
for (const auto& pair : myMap) {
std::cout << pair.first << " => " << pair.second << std::endl;
}
return 0;
}
```
### 解释
- **CustomCompare**:这是一个结构体,重载了 `operator()`,用于定义自定义的比较规则。在这个例子中,我们实现了降序排序。
- **decltype(comp)**:这是 C++11 引入的一个关键字,用于获取变量的类型。在这里,我们用它来推断 lambda 表达式的类型,以便在创建 `std::map` 时使用。
通过这种方式,你可以根据需要自定义 `std::map` 的排序规则。
阅读全文
相关推荐
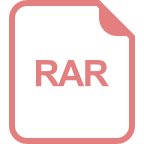
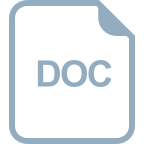






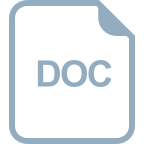
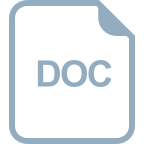
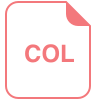







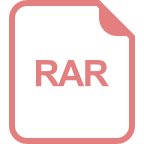