python海龟绘图画沸羊羊
时间: 2023-10-18 16:05:58 浏览: 298
沸羊羊的画像可以使用Python中的海龟绘图库来实现。首先,你需要导入`turtle`模块。然后,你可以使用一系列的`turtle`函数来控制画笔的移动和绘制图形。
下面是一个简单的示例代码,用于绘制沸羊羊的脸部轮廓:
```
import turtle
# 创建一个画布和画笔
canvas = turtle.Screen()
pen = turtle.Turtle()
# 设置画笔的颜色和大小
pen.pensize(4)
pen.color("black")
# 绘制脸部
pen.circle(100)
# 绘制眼睛
pen.penup()
pen.goto(-40, 120)
pen.pendown()
pen.circle(15)
pen.penup()
pen.goto(40, 120)
pen.pendown()
pen.circle(15)
# 绘制嘴巴
pen.penup()
pen.goto(-40, 90)
pen.pendown()
pen.circle(40, 180)
# 绘制牙齿
pen.penup()
pen.goto(-20, 90)
pen.pendown()
pen.setheading(-60)
pen.forward(40)
pen.backward(40)
pen.setheading(-120)
pen.forward(40)
# 隐藏画笔
pen.hideturtle()
# 关闭画布
canvas.exitonclick()
```
这段代码将绘制一个沸羊羊的简单轮廓,包括脸部、眼睛和嘴巴。
相关问题
python绘图画沸羊羊
Python是一种流行的编程语言,它提供了丰富的绘图库,可以用来画各种图形,包括沸羊羊。下面是使用Python绘制沸羊羊的简单示例代码:
```python
import matplotlib.pyplot as plt
# 定义沸羊羊的坐标点
x = [1, 2, 3, 4, 5]
y = [3, 2, 4, 1, 5]
# 绘制沸羊羊
plt.plot(x, y, marker='o')
# 添加标题和坐标轴标签
plt.title("Fei Yangyang")
plt.xlabel("X")
plt.ylabel("Y")
# 显示图形
plt.show()
```
这段代码使用了`matplotlib`库来进行绘图,通过`plot`函数传入沸羊羊的坐标点,然后使用`marker`参数指定点的样式为圆形。最后使用`title`、`xlabel`和`ylabel`函数添加标题和坐标轴标签,再调用`show`函数显示图形。
python用turtle画沸羊羊
以下是使用Python的turtle库画沸羊羊的代码:
```python
import turtle
# 设置画笔初始位置和朝向
turtle.penup()
turtle.goto(-100, 100)
turtle.pendown()
turtle.setheading(-60)
# 画出羊的头部和身体
turtle.begin_fill()
turtle.circle(50, steps=3)
turtle.color('yellow')
turtle.end_fill()
turtle.begin_fill()
turtle.circle(-50, steps=3)
turtle.color('white')
turtle.end_fill()
# 画出羊的脚
turtle.penup()
turtle.goto(-60, 0)
turtle.pendown()
turtle.setheading(-90)
turtle.color('black')
turtle.begin_fill()
turtle.forward(30)
turtle.right(90)
turtle.forward(10)
turtle.right(90)
turtle.forward(30)
turtle.right(90)
turtle.forward(10)
turtle.end_fill()
turtle.penup()
turtle.goto(10, 0)
turtle.pendown()
turtle.setheading(-90)
turtle.color('black')
turtle.begin_fill()
turtle.forward(30)
turtle.right(90)
turtle.forward(10)
turtle.right(90)
turtle.forward(30)
turtle.right(90)
turtle.forward(10)
turtle.end_fill()
# 画出羊的耳朵和眼睛
turtle.penup()
turtle.goto(-90, 120)
turtle.pendown()
turtle.setheading(60)
turtle.color('white')
turtle.begin_fill()
turtle.circle(-20, steps=3)
turtle.end_fill()
turtle.penup()
turtle.goto(-85, 130)
turtle.pendown()
turtle.setheading(0)
turtle.color('black')
turtle.begin_fill()
turtle.circle(-5)
turtle.end_fill()
turtle.penup()
turtle.goto(-60, 120)
turtle.pendown()
turtle.setheading(120)
turtle.color('white')
turtle.begin_fill()
turtle.circle(-20, steps=3)
turtle.end_fill()
turtle.penup()
turtle.goto(-55, 130)
turtle.pendown()
turtle.setheading(0)
turtle.color('black')
turtle.begin_fill()
turtle.circle(-5)
turtle.end_fill()
# 完成画图
turtle.hideturtle()
turtle.done()
```
运行代码后,就可以在turtle窗口里看到画出来的沸羊羊了。
阅读全文
相关推荐

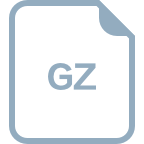














