用 C++ 设计一个名为Rectangle的矩形类,其私有属性为矩形的左上角和右下角两个点的坐标,成员函数能计算和输出矩形的周长,并统计程序中目前存在矩形类对象的个数,然后写一个友元函数求矩形的面积。
时间: 2024-10-14 08:09:29 浏览: 38
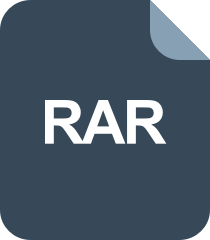
定义一个名为rectangle 的矩形类,其属性数据为矩形左上角和右上角的点的坐标能计算矩形的面积

在C++中,我们可以设计一个名为`Rectangle`的类,用于表示矩形,包含左上角和右下角的坐标,同时也提供必要的功能如计算周长、面积,以及统计对象个数。以下是一个简单的实现:
```cpp
#include <iostream>
#include <vector>
class Point {
public:
int x, y;
Point(int x, int y) : x(x), y(y) {}
};
class Rectangle {
private:
Point topLeft, bottomRight; // 私有属性
static int count; // 计数器
public:
Rectangle(Point tl, Point br) : topLeft(tl), bottomRight(br) {
++count; // 矩形对象增加计数
}
// 计算周长
int perimeter() const {
return 2 * (topLeft.x + bottomRight.x + bottomRight.y - topLeft.y);
}
// 输出矩形周长
void printPerimeter() const {
std::cout << "Perimeter: " << perimeter() << std::endl;
}
// 友元函数,计算面积
friend int area();
// 统计对象个数
static int getRectangleCount() {
return count;
}
// 显示矩形区域
void display() const {
std::cout << "Top Left: (" << topLeft.x << ", " << topLeft.y << ")"
<< ", Bottom Right: (" << bottomRight.x << ", " << bottomRight.y << ")" << std::endl;
}
};
int Rectangle::count = 0; // 初始化矩形对象计数器
// 友元函数实现面积计算
int Rectangle::area() const {
return (bottomRight.x - topLeft.x) * (bottomRight.y - topLeft.y);
}
int main() {
Rectangle rect1(Point(0, 0), Point(5, 4));
rect1.printPerimeter();
rect1.display();
std::cout << "Total rectangles: " << Rectangle::getRectangleCount() << std::endl;
Rectangle rect2(Point(3, 3), Point(7, 7));
rect2.printPerimeter();
rect2.display();
std::cout << "Total rectangles: " << Rectangle::getRectangleCount() << std::endl;
return 0;
}
```
在这个例子中,`Rectangle`类包含两个私有`Point`类型的变量,代表矩形的边界。它有一个友元函数`area()`用于计算面积,一个全局变量`count`用于记录矩形对象的数量,并且提供了`perimeter()`、`printPerimeter()`和`getRectangleCount()`方法。在`main`函数中,我们创建了两个`Rectangle`对象并显示了相关信息。
阅读全文
相关推荐
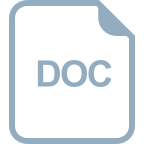
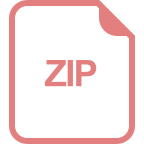
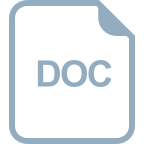
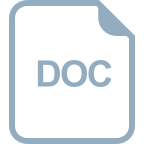
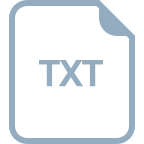
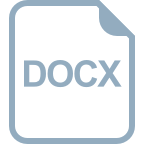
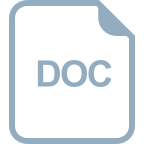
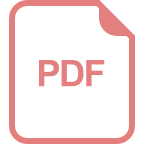
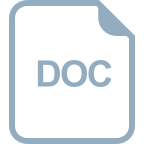
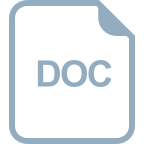
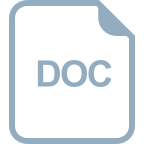

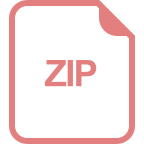