class LSTMNet(torch.nn.Module): def __init__(self, num_hiddens, num_outputs): super(LSTMNet, self).__init__() #nn.Conv1d(1,16,2), #nn.Sigmoid(), # nn.MaxPool1d(2), #nn.Conv1d(1,32,2), self.hidden_size = num_hiddens # RNN 层,这里的 batch_first 指定传入的是 (批大小,序列长度,序列每个位置的大小) # 如果不指定其为 True,传入顺序应当是 (序列长度,批大小,序列每个位置的大小) input_size= num_inputs.view(len(input_x), 1, -1)//24 self.rnn = torch.nn.LSTM(input_size, hidden_size=num_hiddens,batch_first=True) # 线性层 self.dense = torch.nn.Linear(self.hidden_size*24, 256) self.dense2 = torch.nn.Linear(256,num_outputs) # dropout 层,这里的参数指 dropout 的概率 self.dropout = torch.nn.Dropout(0.3) self.dropout2 = torch.nn.Dropout(0.5) # ReLU 层 self.relu = torch.nn.ReLU() # 前向传播函数,这是一个拼接的过程,使用大量变量是为了避免混淆,不做过多讲解 def forward(self, x): # x shape: (batch_size, 24, 307) # LSTM 层会传出其参数,这里用 _ 将其舍弃 h, _ = self.rnn(x) # LSTM 层会传出 (batch_size, 24, num_hiddens) 个参数,故需要 reshape 后丢入全连接层 h_r = h.reshape(-1,self.hidden_size*24) h_d = self.dropout(h_r) y = self.dense(h_d) drop_y = self.dropout2(y) a = self.relu(drop_y) y2 = self.dense2(a) return y2
时间: 2024-04-19 16:30:13 浏览: 199
这是一个使用LSTM网络进行序列预测的模型,输入是一个形状为(batch_size, 24, 307)的张量。在模型的初始化方法中,定义了LSTM层、线性层、dropout层和ReLU层。在前向传播方法中,首先将输入张量传入LSTM层得到输出h,然后将h重新reshape成形状为(batch_size, num_hiddens*24)的张量,再经过线性层、dropout层和ReLU层得到最终的预测结果y2。
相关问题
class NormedLinear(nn.Module): def __init__(self, feat_dim, num_classes): super().__init__() self.weight = nn.Parameter(torch.Tensor(feat_dim, num_classes)) self.weight.data.uniform_(-1, 1).renorm_(2, 1, 1e-5).mul_(1e5) def forward(self, x): return F.normalize(x, dim=1).mm(F.normalize(self.weight, dim=0)) class LearnableWeightScalingLinear(nn.Module): def __init__(self, feat_dim, num_classes, use_norm=False): super().__init__() self.classifier = NormedLinear(feat_dim, num_classes) if use_norm else nn.Linear(feat_dim, num_classes) self.learned_norm = nn.Parameter(torch.ones(1, num_classes)) def forward(self, x): return self.classifier(x) * self.learned_norm class DisAlignLinear(nn.Module): def __init__(self, feat_dim, num_classes, use_norm=False): super().__init__() self.classifier = NormedLinear(feat_dim, num_classes) if use_norm else nn.Linear(feat_dim, num_classes) self.learned_magnitude = nn.Parameter(torch.ones(1, num_classes)) self.learned_margin = nn.Parameter(torch.zeros(1, num_classes)) self.confidence_layer = nn.Linear(feat_dim, 1) torch.nn.init.constant_(self.confidence_layer.weight, 0.1) def forward(self, x): output = self.classifier(x) confidence = self.confidence_layer(x).sigmoid() return (1 + confidence * self.learned_magnitude) * output + confidence * self.learned_margin class MLP_ConClassfier(nn.Module): def __init__(self): super(MLP_ConClassfier, self).__init__() self.num_inputs, self.num_hiddens_1, self.num_hiddens_2, self.num_hiddens_3, self.num_outputs \ = 41, 512, 128, 32, 5 self.num_proj_hidden = 32 self.mlp_conclassfier = nn.Sequential( nn.Linear(self.num_inputs, self.num_hiddens_1), nn.ReLU(), nn.Linear(self.num_hiddens_1, self.num_hiddens_2), nn.ReLU(), nn.Linear(self.num_hiddens_2, self.num_hiddens_3), ) self.fc1 = torch.nn.Linear(self.num_hiddens_3, self.num_proj_hidden) self.fc2 = torch.nn.Linear(self.num_proj_hidden, self.num_hiddens_3) self.linearclassfier = nn.Linear(self.num_hiddens_3, self.num_outputs) self.NormedLinearclassfier = NormedLinear(feat_dim=self.num_hiddens_3, num_classes=self.num_outputs) self.DisAlignLinearclassfier = DisAlignLinear(feat_dim=self.num_hiddens_3, num_classes=self.num_outputs, use_norm=True) self.LearnableWeightScalingLinearclassfier = LearnableWeightScalingLinear(feat_dim=self.num_hiddens_3, num_classes=self.num_outputs, use_norm=True)
这段代码定义了一个名为MLP_ConClassfier的神经网络模型,它包含了多个子模块,包括三个不同的分类器:NormedLinearclassfier、DisAlignLinearclassfier和LearnableWeightScalingLinearclassfier。这些分类器都是基于输入特征进行分类的,并且使用不同的方法来实现分类功能。此外,该模型还包含了一个MLP网络,用于将输入特征映射到更高维的特征空间中。该模型的输入特征维度为41,输出类别数为5。
在执行:class MLP(torch.nn.Module): def __init__(self, weight_decay=0.01): super(MLP, self).__init__() self.fc1 = torch.nn.Linear(178, 100) self.relu = torch.nn.ReLU() self.fc2 = torch.nn.Linear(100, 50) self.fc3 = torch.nn.Linear(50, 5) self.dropout = torch.nn.Dropout(p=0.1) self.weight_decay = weight_decay def forward(self, x): x = self.fc1(x) x = self.relu(x) x = self.fc2(x) x = self.relu(x) x = self.fc3(x) return x def regularization_loss(self): reg_loss = torch.tensor(0.).to(device) for name, param in self.named_parameters(): if 'weight' in name: reg_loss += self.weight_decay * torch.norm(param) return reg_lossmodel = MLP() criterion = torch.nn.CrossEntropyLoss() optimizer = torch.optim.Adam(model.parameters(), lr=0.001) for epoch in range(num_epochs): for i, (inputs, labels) in enumerate(train_loader): optimizer.zero_grad() outputs = model(inputs.to(device)) loss = criterion(outputs, labels.to(device)) loss += model.regularization_loss() loss.backward() optimizer.step()如何将其中测试集的loss函数收敛
到最小值?
首先,需要检查训练集和测试集的loss值是否有明显的差距,如果有,则需要增加模型复杂度或者增加训练集的数量。其次,可以尝试调整learning rate、weight decay等超参数,使模型更容易收敛。另外,可以适当增加训练次数,同时检查是否存在过拟合的情况,控制模型的复杂度,以提高模型的泛化能力。最后,还可以尝试使用更高级的优化算法,如AdamW等。
阅读全文
相关推荐
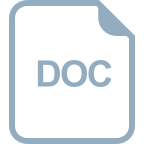
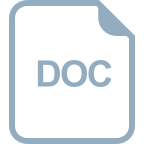
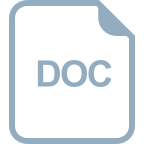













