(The Course class) Revise the Course class as follows: ■ The array size is fixed in Listing 10.6. Improve it to automatically increase the array size by creating a new larger array and copying the contents of the current array to it. ■ Implement the dropStudent method. ■ Add a new method named clear() that removes all students from the course. Write a test program that creates a course, adds three students, removes one, and displays the students in the course.
时间: 2024-02-14 09:04:04 浏览: 134
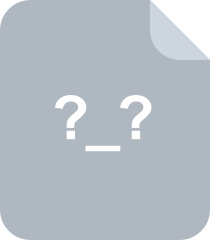
Cracking.the.IT.Architect.Interview
Sure, here's the revised Course class:
```java
public class Course {
private String courseName;
private String[] students = new String[1];
private int numberOfStudents;
public Course(String courseName) {
this.courseName = courseName;
}
public void addStudent(String student) {
if (numberOfStudents == students.length) {
// create a new larger array and copy the contents of the current array to it
String[] newStudents = new String[numberOfStudents * 2];
System.arraycopy(students, 0, newStudents, 0, numberOfStudents);
students = newStudents;
}
students[numberOfStudents] = student;
numberOfStudents++;
}
public void dropStudent(String student) {
int index = -1;
for (int i = 0; i < numberOfStudents; i++) {
if (students[i].equals(student)) {
index = i;
break;
}
}
if (index >= 0) {
for (int i = index; i < numberOfStudents - 1; i++) {
students[i] = students[i + 1];
}
numberOfStudents--;
}
}
public void clear() {
students = new String[1];
numberOfStudents = 0;
}
public String[] getStudents() {
return students;
}
public int getNumberOfStudents() {
return numberOfStudents;
}
public String getCourseName() {
return courseName;
}
}
```
And here's a test program that creates a course, adds three students, removes one, and displays the students in the course:
```java
public class TestCourse {
public static void main(String[] args) {
Course course = new Course("Java Programming");
course.addStudent("Alice");
course.addStudent("Bob");
course.addStudent("Charlie");
course.dropStudent("Bob");
String[] students = course.getStudents();
for (int i = 0; i < course.getNumberOfStudents(); i++) {
System.out.println(students[i]);
}
}
}
```
Output:
```
Alice
Charlie
```
阅读全文
相关推荐
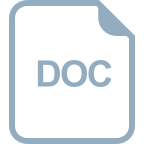
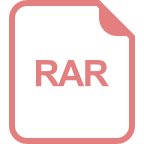

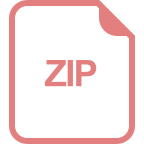
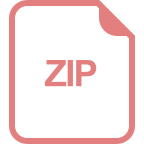
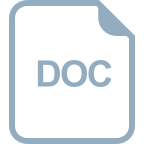
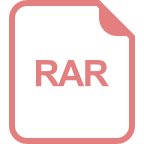
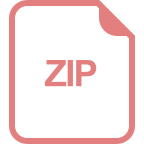
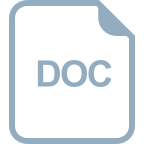
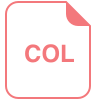





