python与opencv摄像头识别数字代码
时间: 2023-11-19 19:04:32 浏览: 136
你可以使用OpenCV库和Python语言来实现摄像头识别数字的功能。以下是一个示例代码:
```python
import cv2
# 定义数字分类器
digits_classifier = cv2.ml.KNearest_create()
# 加载数字分类器
digits_classifier.load('digits_classifier.xml')
# 定义摄像头对象
cap = cv2.VideoCapture(0)
while True:
# 读取每一帧图像
ret, frame = cap.read()
# 将帧图像转换为灰度图像
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
# 进行图像阈值处理
ret, thresh = cv2.threshold(gray, 0, 255, cv2.THRESH_BINARY_INV + cv2.THRESH_OTSU)
# 查找数字轮廓
contours, hierarchy = cv2.findContours(thresh, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
# 遍历每一个数字轮廓
for cnt in contours:
# 获取数字轮廓的边界框
x, y, w, h = cv2.boundingRect(cnt)
# 获取数字图像
digit = thresh[y:y+h, x:x+w]
# 将数字图像缩放到相同大小
resized_digit = cv2.resize(digit, (20, 20))
# 将数字图像转换为一维数组
digit_data = resized_digit.reshape(-1)
# 将一维数组转换为单行矩阵
digit_matrix = np.float32(digit_data).reshape((1, -1))
# 使用数字分类器对数字图像进行分类
ret, result, neighbors, dist = digits_classifier.findNearest(digit_matrix, k=1)
# 显示识别结果
cv2.putText(frame, str(int(result)), (x, y), cv2.FONT_HERSHEY_SIMPLEX, 1, (0, 255, 0), 2)
# 显示帧图像
cv2.imshow('frame', frame)
# 等待按下 Esc 键退出
if cv2.waitKey(1) == 27:
break
# 释放摄像头对象
cap.release()
# 关闭所有窗口
cv2.destroyAllWindows()
```
运行代码后,摄像头会自动打开,并且能够实时识别出摄像头画面中的数字并显示在窗口上。
阅读全文
相关推荐


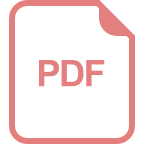
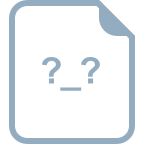
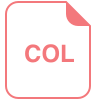




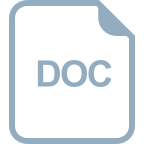
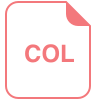
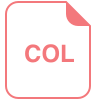
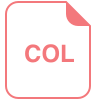

