//动态链表 #include "stdio.h" #include "stdlib.h" #define LEN sizeof(struct Student) struct Student{ long num; float score; struct Student *next; }; int n; //全局变量 struct Student*creat(void) //建立链表 { //此函数无需放入形参 struct Student *head ,*p1,*p2; n=0; p1=p2=(struct Student *)malloc(LEN); scanf("%ld%f",&p1->num,&p1->score); while(p1->num!=0) //此处判断遇 0 代表结束 { n=n+1; if(n==1) head=p1; else p2->next=p1; p2=p1; p1=(struct Student *) malloc(LEN); scanf("%ld%f",&p1->num,&p1->score); } p2->next=NULL; return head; //返回值是链表头 } void del(struct Student *head) //删除结点 { struct Student *p1,*p2,*p; int k,a=n; printf("请输入要删除的结点:\n"); scanf("%d",&k); p1=head; while(n==a&&p1->next!=NULL) { if(head->num==k) //删除的是头结点 { p1=head; // 原头结点 p2= p1->next; // 新的头结点 free(p1); // 释放原头结点内存空间 head =p2; // 头指针向后移一位 n-=1; } p2=p1; p1=p1->next; if(p1->num==k) { p=p1->next; p2->next=p; n-=1; } } } void print(struct Student *head)//输出链表 { struct Student *p; p=head; printf("\nNow,These %d reconds are:\n",n); if(head!=NULL) do { printf("%ld %5.1f\n",p->num,p->score); p=p->next; }while(p!=NULL); } int main() { struct Student *pt; pt=creat(); del(pt); print(pt); return 0; }哪里有错误
时间: 2024-02-14 21:12:02 浏览: 30
代码中存在一个潜在的错误。当删除的是最后一个结点时,程序会陷入死循环,无法退出。具体来说,是由于在while循环中,当p1指向最后一个结点时,p1->next为NULL,但是判断条件却是p1->next!=NULL,导致循环无法结束。应该将判断条件改为p1!=NULL,这样当p1指向最后一个结点时,循环可以正常退出。修改后的代码如下:
```
void del(struct Student *head) //删除结点
{
struct Student *p1,*p2,*p;
int k,a=n;
printf("请输入要删除的结点:\n");
scanf("%d",&k);
p1=head;
while(n==a&&p1!=NULL)
{
if(head->num==k) //删除的是头结点
{
p1=head; // 原头结点
p2= p1->next; // 新的头结点
free(p1); // 释放原头结点内存空间
head =p2; // 头指针向后移一位
n-=1;
}
p2=p1;
p1=p1->next;
if(p1!=NULL && p1->num==k)
{
p=p1->next;
p2->next=p;
n-=1;
}
}
}
```
相关推荐
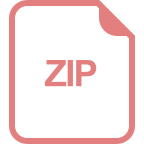
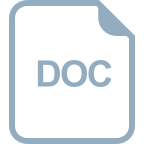
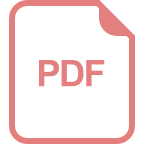














