创建和输出链表 函数create()的功能是:建立动态链表,返回链表头指针head,要求从键盘输入各结点的数据,当输入值为0时停止结点的建立。print函数输出链表head中各个结点的data值。请将create函数和print函数补充完整。 输入格式: 在一行输入结点数据,以空格间隔,最后一个输入0,代表输入结束(0不成为新的结点)。 输出格式: 输出链表的每个结点的数据域,每个数据后有一个空格,最后换行,注意,如为空链表,输出就是一个换行。 输入样例: 在这里给出一组输入。例如: 1 2 3 4 5 6 0 输出样例: 在这里给出相应的输出。例如: 1 2 3 4 5 6 可在如下程序中补充代码,也可自行编写代码。 #include <stdio.h> #include <stdlib.h> #define LEN sizeof(nod) typedef struct node { int data; struct node *next; }nod; nod *create() { /**/ /**/ } void print(nod *head) { /**/ /**/ } int main() { nod *head; head=/**/ /**/ //调用create函数 /**/ /**/ //调用print函数 return 0; }
时间: 2024-02-16 17:03:13 浏览: 64
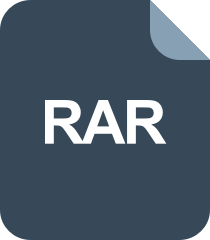
链表的创建和输出
以下是完整代码:
```c
#include <stdio.h>
#include <stdlib.h>
#define LEN sizeof(nod)
typedef struct node {
int data;
struct node *next;
} nod;
nod *create() {
nod *head, *p1, *p2;
int n;
head = NULL;
p1 = p2 = (nod *) malloc(LEN);
scanf("%d", &n);
while (n != 0) {
p1->data = n;
if (head == NULL) {
head = p1;
} else {
p2->next = p1;
}
p2 = p1;
p1 = (nod *) malloc(LEN);
scanf("%d", &n);
}
p2->next = NULL;
return head;
}
void print(nod *head) {
nod *p;
p = head;
if (head == NULL) {
printf("\n");
return;
}
while (p != NULL) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
}
int main() {
nod *head;
head = create();
print(head);
return 0;
}
```
阅读全文
相关推荐











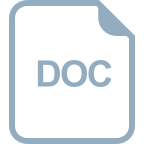
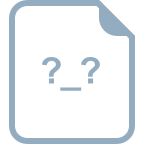
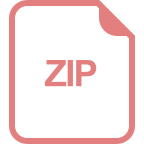
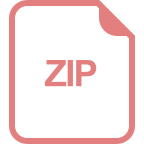