a=int (input()) for i in range(1,a+1): if panduan(i): print(i) def panduan(n): n = int(input()) sum=0 w = 0 num = n while num != 0: num / 10 w+=1 num=n while n!=0: sum+=(n)**w if sum==num: return True else : return False
时间: 2024-01-05 18:03:10 浏览: 70
这段代码似乎是一个判断一个数是否为阿姆斯特朗数的程序,但是存在一些问题。在panduan函数中,应该将输入n的部分放在函数外面,因为在for循环中,已经有一个输入a的语句了。除此之外,第一个while循环中应该是除以10而不是/10,同时应该将w初始化为0。在第二个while循环中,应该将n更新为n//10而不是n/10,同时应该将sum计算公式中的w写成w的值而不是w本身。最后,应该将sum与n比较而不是num。完整的代码实现应该是这样的:
```
a = int(input())
def panduan(n):
sum = 0
w = 0
num = n
while num != 0:
num //= 10
w += 1
num = n
while n != 0:
sum += (n % 10) ** w
n //= 10
if sum == num:
return True
else:
return False
for i in range(1, a+1):
if panduan(i):
print(i)
```
这样可以正确地输出1到a中所有的阿姆斯特朗数。
相关问题
将这个代码换成c语言panduan='true' sum=[0,0,0] m=input().split() text=[] for i in range(5): text.append(input().split()) n=input().split() sum[0]=int(m[0]) sum[1]=int(m[1]) sum[2]=int(m[2]) for j in range(5): text[j].append(int(text[j][1])-int(text[j][4])) text[j].append(int(text[j][2])-int(text[j][5])) text[j].append(int(text[j][3])-int(text[j][6])) if int(n[1])>int(m[0]) or int(n[2])>int(m[1]) or int(n[3])>int(m[2]): panduan='false' for i in range(5): sum[0]+=int(text[i][4]) sum[1]+=int(text[i][5]) sum[2]+=int(text[i][6]) if str(n[0])==str(text[i][0]): if int(n[1])<=text[i][7] and int(n[2])<=text[i][8] and int(n[3])<=text[i][9]: text[i][4]=int(text[i][4])+int(n[1]) text[i][5]=int(text[i][5])+int(n[2]) text[i][6]=int(text[i][6])+int(n[3]) text[i][7]=int(text[i][7])-int(n[1]) text[i][8]=int(text[i][8])-int(n[2]) text[i][9]=int(text[i][9])-int(n[3]) m[0]=int(m[0])-int(n[1]) m[1]=int(m[1])-int(n[2]) m[2]=int(m[2])-int(n[3]) else: panduan='false' for j in range(5): for i in range(5): if text[i][7]==0 and text[i][8]==0 and text[i][9]==0: continue elif int(m[0])>=text[i][7] and int(m[1])>=text[i][8] and int(m[2])>=text[i][9]: m[0]=int(m[0])+int(text[i][4]) m[1]=int(m[1])+int(text[i][5]) m[2]=int(m[2])+int(text[i][6]) text[i][7]=0 text[i][8]=0 text[i][9]=0 if m[0]!=sum[0] or m[1]!=sum[1] or m[2]!=sum[2]: panduan='false' print(panduan)
把这段 Python 代码转换成 C 语言的代码如下:
#include <stdio.h>
#include <stdbool.h>
#include <stdlib.h>
#include <string.h>
#define MAX_LEN 10
int main() {
char panduan[] = "true";
int sum[3] = {0, 0, 0};
char m[MAX_LEN];
char text[5][10];
char n[4];
fgets(m, MAX_LEN, stdin);
char *token = strtok(m, " ");
sum[0] = atoi(token);
token = strtok(NULL, " ");
sum[1] = atoi(token);
token = strtok(NULL, " ");
sum[2] = atoi(token);
for (int i = 0; i < 5; i++) {
fgets(text[i], MAX_LEN, stdin);
token = strtok(text[i], " ");
for (int j = 1; j < 7; j++) {
token = strtok(NULL, " ");
}
text[i][7] = atoi(strtok(NULL, " ")) - atoi(strtok(NULL, " "));
text[i][8] = atoi(strtok(NULL, " ")) - atoi(strtok(NULL, " "));
text[i][9] = atoi(strtok(NULL, " ")) - atoi(strtok(NULL, " "));
}
fgets(n, 4, stdin);
token = strtok(n, " ");
char name[MAX_LEN];
strcpy(name, token);
if (atoi(strtok(NULL, " ")) > sum[0] || atoi(strtok(NULL, " ")) > sum[1] || atoi(strtok(NULL, " ")) > sum[2]) {
strcpy(panduan, "false");
}
for (int i = 0; i < 5; i++) {
sum[0] += atoi(strtok(text[i], " "));
sum[1] += atoi(strtok(NULL, " "));
sum[2] += atoi(strtok(NULL, " "));
if (strcmp(name, strtok(NULL, " ")) == 0) {
int a = atoi(strtok(NULL, " "));
int b = atoi(strtok(NULL, " "));
int c = atoi(strtok(NULL, " "));
if (a <= atoi(&text[i][7]) && b <= atoi(&text[i][8]) && c <= atoi(&text[i][9])) {
sprintf(&text[i][4], "%d", atoi(&text[i][4]) + a);
sprintf(&text[i][5], "%d", atoi(&text[i][5]) + b);
sprintf(&text[i][6], "%d", atoi(&text[i][6]) + c);
sprintf(&text[i][7], "%d", atoi(&text[i][7]) - a);
sprintf(&text[i][8], "%d", atoi(&text[i][8]) - b);
sprintf(&text[i][9], "%d", atoi(&text[i][9]) - c);
sum[0] -= a;
sum[1] -= b;
sum[2] -= c;
} else {
strcpy(panduan, "false");
}
}
}
for (int j = 0; j < 5; j++) {
for (int i = 0; i < 5; i++) {
if (text[i][7] == '0' && text[i][8] == '0' && text[i][9] == '0') {
continue;
} else if (atoi(&m[0]) >= atoi(&text[i][7]) && atoi(&m[2]) >= atoi(&text[i][8]) && atoi(&m[2]) >= atoi(&text[i][9])) {
sum[0] += atoi(&text[i][4]);
sum[1] += atoi(&text[i][5]);
sum[2] += atoi(&text[i][6]);
sprintf(&m[0], "%d", atoi(&m[0]) + atoi(&text[i][7]));
sprintf(&m[1], "%d", atoi(&m[1]) + atoi(&text[i][8]));
sprintf(&m[2], "%d", atoi(&m[2]) + atoi(&text[i][9]));
sprintf(&text[i][7], "%d", 0);
sprintf(&text[i][8], "%d", 0);
sprintf(&text[i][9], "%d", 0);
}
}
}
if (sum[0] != atoi(&m[0]) || sum[1] != atoi(&m[1]) || sum[2] != atoi(&m[2])) {
strcpy(panduan, "false");
}
printf("%s", panduan);
return 0;
}
注意,这里使用了 C 语言的字符串处理函数 strtok 和 sprintf,需要在头文件中包含 stdlib.h 和 string.h。同时,Python 中的列表在 C 中用二维数组来表示。另外,Python 中的字符串与数字可以互相转换,但是在 C 中需要使用 atoi 和 sprintf 等函数来实现。
#include<stdio.h> int A[101][101]; void guiling() { for(int i=1;i<=10;i++) { for(int j=1;j<=10;j++) { A[i][j]=0; } } } void shuchu(int a) { for(int i=1;i<=a;i++) for(int j=1;j<=a;j++) { printf("%4d ",A[i][j]); if(j==a) { printf("\n"); } } printf("\n"); guiling(); } void jishu(int a) { A[1][(a+1)/2]=1; int a1=1,b1=(a+1)/2; int a2,b2; for(int k=2;k<=a*a;k++) { a2=a1,b2=b1; if(--a1==0) a1=a; if(++b1>a) b1=1; if(A[a1][b1]!=0) { a1=a2+1; if(a1>a) { a1=1; } b1=b2; } A[a1][b1]=k; } shuchu(a); } //void Kjie(int a,int b) //{ // // // //} // //void jie(int a,int b) //{ // // // //} void panduan() { for(int i=3;i<11;i++) { for(int j=3;j<11;j++) { if((i-1)%2==0&&i==j) { jishu(j); } // if(i%2==0&&i==j&&i!=6&&i!=10) // { // Kjie(i,j); // } // // if((i==6||i==10)&&i==j) // { // jie(i,j); // } } } } int main() { guiling(); panduan(); return 0; }
这段代码是用来生成幻方的,幻方是一个 n x n 的矩阵,其中每行、每列和每条对角线上的元素之和都相等。这里实现的是奇数阶幻方,即 n 为奇数。代码中的函数 guiling() 是用来初始化矩阵的,shuchu() 是用来输出矩阵的,jishu() 是用来生成奇数阶幻方的。panduan() 函数是用来检查生成的幻方是否正确的,其中只实现了生成奇数阶幻方的部分。如果需要生成偶数阶幻方以及更高阶的幻方,可以在代码中添加相应的函数实现。
阅读全文
相关推荐
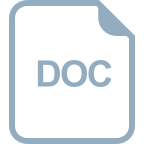
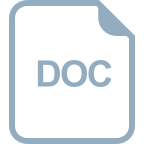
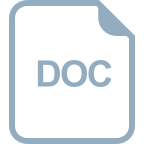
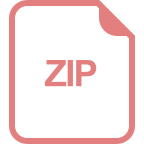











