Linux 串口接收函数
时间: 2024-12-29 21:12:11 浏览: 5
### Linux 串口通信接收函数示例
在Linux环境中,`termios` 结构体提供了对串行端口的控制功能。为了实现串口通信中的数据接收,通常会结合 `read()` 或者更高级别的 I/O 多路复用机制如 `poll()` 和 `select()` 来处理输入输出操作。
#### 使用 read() 进行简单读取
当使用 `read()` 函数来从已配置好的串行端口中获取数据时,可以按照下面的方式编写代码:
```c
#include <stdio.h>
#include <unistd.h> /* For close */
#include <fcntl.h> /* File control definitions */
#include <errno.h> /* Error number definitions */
#include <termios.h> /* POSIX terminal control definitions */
int main()
{
int fd;
char buf[256];
// 打开串行端口
fd = open("/dev/ttyS0", O_RDWR | O_NOCTTY);
if (fd < 0){
perror("Opening serial port");
return -1;
}
// 配置 termios 参数...
struct termios options;
tcgetattr(fd, &options);
cfsetispeed(&options, B9600);
cfsetospeed(&options, B9600);
options.c_cflag |= (CLOCAL | CREAD);
options.c_cflag &= ~PARENB;
options.c_cflag &= ~CSTOPB;
options.c_cflag &= ~CSIZE;
options.c_cflag |= CS8;
tcsetattr(fd, TCSANOW, &options);
// 尝试读取数据
ssize_t bytes_read = read(fd, buf, sizeof(buf)-1);
if(bytes_read > 0){
buf[bytes_read]='\0';
printf("Received data: %s\n", buf);
}else {
fprintf(stderr,"Error reading from device (%d)\n", errno);
}
close(fd);
}
```
这段代码展示了如何设置并打开一个串行端口 `/dev/ttyS0` ,然后尝试从中读取最多 255 字节的数据到缓冲区中[^1]。
#### 利用 poll() 实现非阻塞读取
对于需要高效管理多个文件描述符的应用场景来说,采用 `poll()` 可以有效提高性能。这里给出一段利用 `poll()` 的例子来进行非阻塞性质的数据接收:
```c
#include <poll.h>
// ... 前面部分保持不变 ...
struct pollfd fds[1];
fds[0].fd = fd;
fds[0].events = POLLIN;
while(1){
int ret = poll(fds, 1, -1);
if(ret == -1){
perror("Poll error");
break;
}
else if(ret && (fds[0].revents&POLLIN)){
ssize_t bytes_read = read(fd,buf,sizeof(buf));
if(bytes_read > 0){
buf[bytes_read]='\0';
printf("Data received:%s\n",buf);
}
}
}
// 关闭串口连接 ...
close(fd);
```
此段程序会在每次检测到有可读事件发生时立即去读取消息内容,并打印出来直到遇到错误为止[^3].
阅读全文
相关推荐




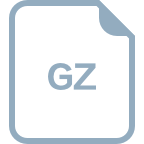













