Linux 串口接收不定长度的数据
时间: 2023-11-21 10:04:04 浏览: 353
在 Linux 中,串口接收不定长度的数据是一个常见的问题。你可以使用以下方法来解决这个问题:
1. 使用阻塞模式读取串口数据:在阻塞模式下,读取串口数据时,程序会一直等待直到接收到指定长度的数据或者超时。你可以使用`read()`函数来读取串口数据,并设置合适的超时时间。例如:
```c
#include <fcntl.h>
#include <termios.h>
#include <unistd.h>
int main() {
int fd = open("/dev/ttyS0", O_RDWR | O_NOCTTY);
// 设置串口参数
struct termios options;
tcgetattr(fd, &options);
// 根据需求设置波特率、数据位、停止位等参数
cfsetispeed(&options, B9600);
cfsetospeed(&options, B9600);
tcsetattr(fd, TCSANOW, &options);
char buffer[255];
int bytesRead = read(fd, buffer, sizeof(buffer));
if (bytesRead > 0) {
// 处理接收到的数据
// ...
}
close(fd);
return 0;
}
```
2. 使用非阻塞模式读取串口数据:在非阻塞模式下,读取串口数据时,程序会立即返回,不管是否有数据可读。你可以使用`select()`函数来检查串口是否有可读数据,并使用`read()`函数来读取数据。例如:
```c
#include <fcntl.h>
#include <termios.h>
#include <unistd.h>
#include <sys/select.h>
int main() {
int fd = open("/dev/ttyS0", O_RDWR | O_NOCTTY | O_NONBLOCK);
// 设置串口参数
struct termios options;
tcgetattr(fd, &options);
// 根据需求设置波特率、数据位、停止位等参数
cfsetispeed(&options, B9600);
cfsetospeed(&options, B9600);
tcsetattr(fd, TCSANOW, &options);
fd_set readfds;
FD_ZERO(&readfds);
FD_SET(fd, &readfds);
struct timeval timeout;
timeout.tv_sec = 1; // 设置超时时间为1秒
timeout.tv_usec = 0;
char buffer[255];
int ready = select(fd + 1, &readfds, NULL, NULL, &timeout);
if (ready > 0 && FD_ISSET(fd, &readfds)) {
int bytesRead = read(fd, buffer, sizeof(buffer));
if (bytesRead > 0) {
// 处理接收到的数据
// ...
}
}
close(fd);
return 0;
}
```
这些示例代码假设你的串口设备文件是`/dev/ttyS0`,你需要根据实际情况进行更改。另外,记得在编译时链接`-lrt`库以使用`select()`函数。
请注意,以上代码只是演示如何读取串口数据,你还需要根据实际需求来处理接收到的数据。
阅读全文
相关推荐
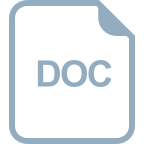
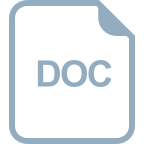
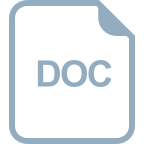
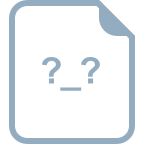
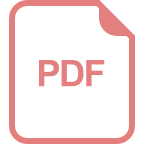
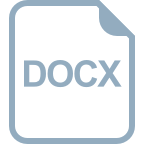
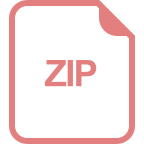
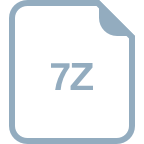
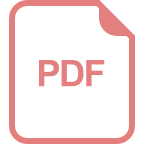
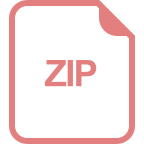
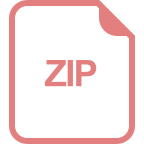
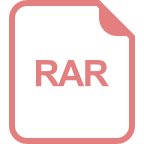
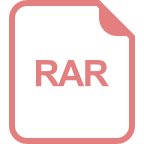
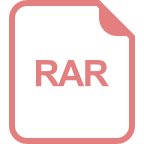
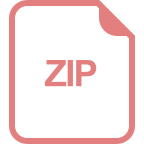
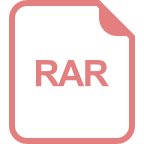