Linux C语言实现串口接收任意长度的数据
时间: 2023-07-27 17:06:38 浏览: 191
要在 Linux 中使用 C 语言实现串口接收任意长度的数据,你可以使用以下代码作为参考:
```c
#include <stdio.h>
#include <fcntl.h>
#include <termios.h>
#include <unistd.h>
int main() {
int fd = open("/dev/ttyS0", O_RDWR | O_NOCTTY);
if (fd == -1) {
perror("Open serial port failed");
return 1;
}
struct termios options;
tcgetattr(fd, &options);
cfsetispeed(&options, B9600); // 设置波特率为9600
cfsetospeed(&options, B9600);
options.c_cflag |= (CLOCAL | CREAD); // 必要的控制标志
options.c_cflag &= ~PARENB; // 禁用奇偶校验
options.c_cflag &= ~CSTOPB; // 1位停止位
options.c_cflag &= ~CSIZE; // 清除数据位设置
options.c_cflag |= CS8; // 设置数据位为8位
tcsetattr(fd, TCSANOW, &options);
char buffer[255]; // 接收缓冲区
int bytesRead = 0; // 已读取字节数
int totalBytesRead = 0; // 总共读取字节数
while (1) {
bytesRead = read(fd, buffer + totalBytesRead, sizeof(buffer) - totalBytesRead);
if (bytesRead > 0) {
totalBytesRead += bytesRead;
// 检查是否接收到完整的数据,可以根据自己的需求进行处理
if (buffer[totalBytesRead - 1] == '\n') {
// 处理接收到的数据
buffer[totalBytesRead] = '\0'; // 添加字符串结尾符
printf("Received: %s", buffer);
totalBytesRead = 0; // 重置总共读取字节数
}
}
}
close(fd);
return 0;
}
```
这段代码打开了串口设备文件`/dev/ttyS0`(根据你的实际情况可能需要修改)并设置了串口参数,如波特率、数据位、停止位等。然后,通过循环不断地读取串口数据。
在每次读取数据后,我们检查是否接收到完整的数据。这里的判断是根据接收到的数据中是否包含换行符('\n')来确定的。如果接收到了完整的数据,我们可以对其进行处理,例如打印出来。然后,重置总共读取字节数,以准备接收下一段数据。
请注意,在实际应用中,你可能需要根据自己的需求对代码进行适当的修改和优化。同时,还应该处理错误和异常情况,以确保程序的稳定性和可靠性。
希望这个例子可以帮助你实现串口接收任意长度的数据。如有任何疑问,请随时提问。
阅读全文
相关推荐
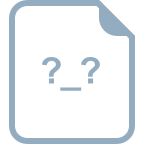
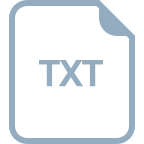
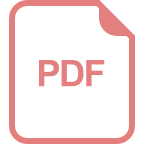
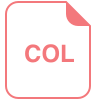
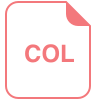
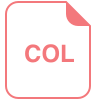
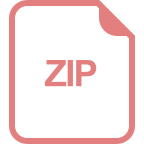
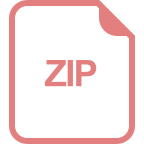
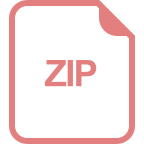
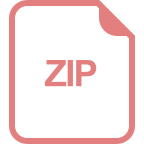
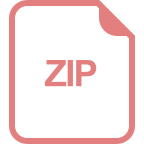