Linux c语言实现串口一次收多个字符程序示例
时间: 2024-09-12 16:06:13 浏览: 49
在Linux环境下,使用C语言编写串口通信程序来接收多个字符通常涉及到`stdio.h`、`fcntl.h`、`termios.h`以及可能需要的特定头文件,如针对某个串口驱动(例如libftdi或libusbx)的头文件。
下面是一个简单的示例,假设你正在使用标准的UART设备,并非特定库:
```c
#include <stdio.h>
#include <fcntl.h>
#include <termios.h>
#define SERIAL_PORT "/dev/ttyUSB0" // 替换为你实际的串口号
int main() {
int fd = -1; // 文件描述符
struct termios options;
// 打开串口
if ((fd = open(SERIAL_PORT, O_RDWR | O_NOCTTY | O_NDELAY)) == -1) {
perror("Opening serial port failed");
return 1;
}
// 初始化控制选项
tcgetattr(fd, &options);
// 设置non-blocking模式
cfsetispeed(&options, B9600); // 设定波特率
cfsetospeed(&options, B9600);
options.c_cflag &= ~CRTSCTS; // 取消硬件流控
options.c_cflag |= CLOCAL | CREAD; // 非控制模式,允许读取
options.c_iflag &= ~(IGNBRK | BRKINT | PARMRK | ISTRIP | INLCR | IGNCR | ICRNL); // 关闭特殊处理
options.c_oflag &= ~OPOST; // 不发送回显
options.c_lflag &= ~(ECHO | ICANON | ISIG); // 关闭输入回显和终端信号
tcflush(fd, TCIFLUSH); // 清空输入缓冲区
// 应用设置
tcsetattr(fd, TCSANOW, &options);
char buffer[256]; // 接收数据的缓冲区
ssize_t bytes_received;
while (true) { // 循环接收直到有数据到达
bytes_received = read(fd, buffer, sizeof(buffer));
if (bytes_received > 0) {
printf("Received %zd characters:\n", bytes_received);
for (size_t i = 0; i < bytes_received; ++i) {
printf("%c ", buffer[i]);
}
printf("\n");
} else if (bytes_received == 0) {
break; // 如果接收到EOF,表示连接关闭
} else {
perror("Reading from serial port failed");
break;
}
}
close(fd);
return 0;
}
```
阅读全文
相关推荐
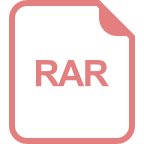
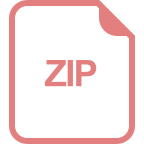
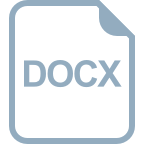















