帮我用c语言写一个在linux环境下实现uart串口读取gps数据的程序
时间: 2024-12-13 11:27:41 浏览: 5
在Linux环境下使用C语言编写UART串口读取GPS数据的程序需要涉及几个关键步骤:包括硬件初始化、设置串口参数、数据接收以及处理GPS解析。以下是一个简化版的示例,假设你已经了解了基本的C编程和Linux系统编程,并且GPS数据是以固定格式发送的:
```c
#include <stdio.h>
#include <fcntl.h>
#include <termios.h>
#include <unistd.h>
#include <sys/ioctl.h>
#define GPS_PORT "/dev/ttyS0" // 替换为实际的GPS串口路径
int main() {
int uart_fd = -1;
struct termios options;
// 打开串口
if ((uart_fd = open(GPS_PORT, O_RDWR | O_NOCTTY | O_NDELAY)) == -1) {
perror("Error opening UART");
return -1;
}
// 设置串口模式
memset(&options, 0, sizeof(options));
cfsetispeed(&options, B9600); // 设置波特率,比如B9600或其他实际速度
cfsetospeed(&options, B9600);
tcflush(uart_fd, TCIFLUSH);
tcgetattr(uart_fd, &options);
options.c_cflag &= ~CSIZE; // 清除数据位宽度标志
options.c_cflag |= CS8; // 设置8位数据
options.c_cflag &= ~(PARENB | PARODD); // 关闭校验位,无奇偶校验
options.c_cflag &= ~CSTOPB; // 单位停止位
options.c_cflag &= ~CRTSCTS; // 不启用RTS/CTS握手
options.c_iflag &= ~(IXON | IXOFF | IGNPAR); // 禁止硬件流控,忽略填充字符
options.c_oflag &= ~OPOST; // 阻塞输出
tcflush(uart_fd, TCIOFLUSH);
tcsetattr(uart_fd, TCSANOW, &options);
char buffer[128]; // 假设GPS数据包最大长度为128字节
while (1) { // 无限循环接收数据
ssize_t bytes_read = read(uart_fd, buffer, sizeof(buffer));
if (bytes_read > 0) {
printf("Received: %s", buffer); // 输出接收到的数据
// 这里应该添加对GPS数据的解析部分,因为GPS数据通常包含特定的标识符(如"$GPRMC")
// 例如,如果数据首字符是'$',可以检查是否开始一个新的数据包
if (buffer[0] == '$') {
parse_gps_data(buffer);
}
} else if (bytes_read < 0 && errno != EAGAIN) {
perror("Error reading from UART");
}
}
close(uart_fd);
return 0;
}
void parse_gps_data(char* data) {
// 这里仅做简单字符串分割,实际解析应包含更复杂的正则表达式或GPS解析库
// 假设GPS数据分隔符是'\n'
size_t pos = 0;
while ((pos = strchr(data + pos, '\n')) != NULL) {
printf("Parsed: %.*s\n", pos - data, data);
pos++; // 跳过换行符
}
}
// 相关问题:
阅读全文
相关推荐
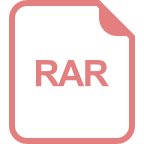
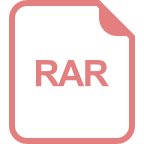
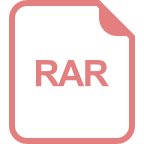
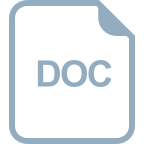
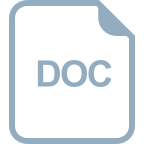


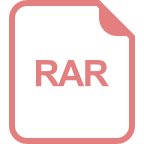
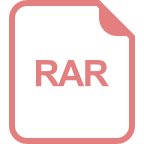
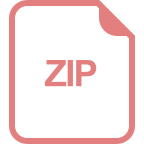
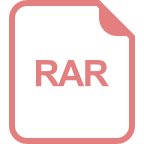
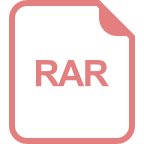
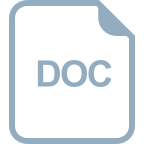
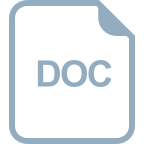
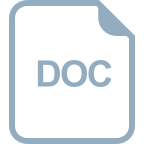



