c++基于ffmpeg创建rtsp服务器
时间: 2024-06-09 16:07:28 浏览: 23
在使用 C++ 基于 FFmpeg 创建 RTSP 服务器之前,需要先了解一些基本概念:
1. RTSP(Real Time Streaming Protocol),实时流传输协议,是一种基于客户端/服务器模型的应用层协议,用于控制实时数据的传输。
2. FFmpeg 是一套开源的音视频编解码库,可以实现音视频的采集、编解码、转换、播放等功能。
3. 在 FFmpeg 中,使用 libavformat 库来处理各种音视频封装格式,例如 MP4、FLV、AVI、MKV、RTMP 等。
4. FFmpeg 中使用 libavcodec 库来处理音视频编解码,例如 H.264、H.265、AAC、MP3 等。
接下来,我们可以按照以下步骤来创建 RTSP 服务器:
1. 初始化 FFmpeg 库,包括注册所有的编解码器、格式和网络协议等。
```c++
av_register_all();
avformat_network_init();
```
2. 创建 AVFormatContext 对象,用于处理音视频封装格式,例如 RTSP、RTMP 等。
```c++
AVFormatContext *pFormatCtx = avformat_alloc_context();
```
3. 打开输入流,例如打开摄像头、麦克风等,或者打开本地文件进行转码。
```c++
AVInputFormat *pInputFormat = av_find_input_format("video4linux2");
if (avformat_open_input(&pFormatCtx, "/dev/video0", pInputFormat, NULL) < 0) {
printf("Could not open input stream\n");
return -1;
}
```
4. 查找视频流和音频流,并且获取相应的编解码器。
```c++
int videoStreamIndex = -1;
int audioStreamIndex = -1;
for (int i = 0; i < pFormatCtx->nb_streams; i++) {
AVCodecParameters *pCodecParameters = pFormatCtx->streams[i]->codecpar;
AVCodec *pCodec = avcodec_find_decoder(pCodecParameters->codec_id);
if (pCodec == NULL) {
printf("Unsupported codec!\n");
continue;
}
if (pCodecParameters->codec_type == AVMEDIA_TYPE_VIDEO) {
videoStreamIndex = i;
} else if (pCodecParameters->codec_type == AVMEDIA_TYPE_AUDIO) {
audioStreamIndex = i;
}
}
```
5. 打开视频流和音频流的编解码器,并且分配相应的 AVCodecContext 对象。
```c++
AVCodecParameters *pVideoCodecParameters = pFormatCtx->streams[videoStreamIndex]->codecpar;
AVCodec *pVideoCodec = avcodec_find_decoder(pVideoCodecParameters->codec_id);
AVCodecContext *pVideoCodecCtx = avcodec_alloc_context3(pVideoCodec);
avcodec_parameters_to_context(pVideoCodecCtx, pVideoCodecParameters);
avcodec_open2(pVideoCodecCtx, pVideoCodec, NULL);
AVCodecParameters *pAudioCodecParameters = pFormatCtx->streams[audioStreamIndex]->codecpar;
AVCodec *pAudioCodec = avcodec_find_decoder(pAudioCodecParameters->codec_id);
AVCodecContext *pAudioCodecCtx = avcodec_alloc_context3(pAudioCodec);
avcodec_parameters_to_context(pAudioCodecCtx, pAudioCodecParameters);
avcodec_open2(pAudioCodecCtx, pAudioCodec, NULL);
```
6. 创建 AVFormatContext 对象,用于输出 RTSP 流。
```c++
AVOutputFormat *pOutputFormat = av_guess_format("rtsp", NULL, NULL);
AVFormatContext *pOutputFormatCtx = avformat_alloc_context();
pOutputFormatCtx->oformat = pOutputFormat;
```
7. 添加视频流和音频流到输出流中,并且设置相应的参数。
```c++
AVStream *pVideoStream = avformat_new_stream(pOutputFormatCtx, NULL);
avcodec_parameters_copy(pVideoStream->codecpar, pVideoCodecParameters);
pVideoStream->codecpar->codec_tag = 0;
AVStream *pAudioStream = avformat_new_stream(pOutputFormatCtx, NULL);
avcodec_parameters_copy(pAudioStream->codecpar, pAudioCodecParameters);
pAudioStream->codecpar->codec_tag = 0;
avio_open(&pOutputFormatCtx->pb, "rtsp://127.0.0.1:8554/test", AVIO_FLAG_WRITE);
avformat_write_header(pOutputFormatCtx, NULL);
```
8. 开始读取输入流,并且将音视频数据写入输出流。
```c++
AVPacket packet;
while (av_read_frame(pFormatCtx, &packet) >= 0) {
if (packet.stream_index == videoStreamIndex) {
avcodec_send_packet(pVideoCodecCtx, &packet);
AVFrame *pFrame = av_frame_alloc();
while (avcodec_receive_frame(pVideoCodecCtx, pFrame) == 0) {
// 处理视频帧数据
avformat_write_frame(pOutputFormatCtx, &packet);
}
av_frame_free(&pFrame);
} else if (packet.stream_index == audioStreamIndex) {
avcodec_send_packet(pAudioCodecCtx, &packet);
AVFrame *pFrame = av_frame_alloc();
while (avcodec_receive_frame(pAudioCodecCtx, pFrame) == 0) {
// 处理音频帧数据
avformat_write_frame(pOutputFormatCtx, &packet);
}
av_frame_free(&pFrame);
}
av_packet_unref(&packet);
}
```
9. 最后,释放所有资源。
```c++
avformat_close_input(&pFormatCtx);
avformat_free_context(pFormatCtx);
avcodec_close(pVideoCodecCtx);
avcodec_free_context(&pVideoCodecCtx);
avcodec_close(pAudioCodecCtx);
avcodec_free_context(&pAudioCodecCtx);
avio_close(pOutputFormatCtx->pb);
avformat_free_context(pOutputFormatCtx);
```
以上就是基于 FFmpeg 创建 RTSP 服务器的一些基本步骤,需要根据具体的需求进行调整和优化。
相关推荐
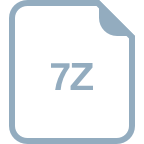






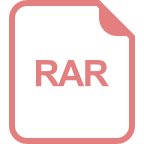
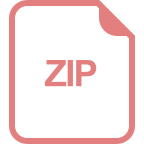
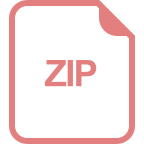
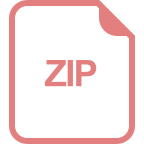
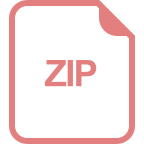
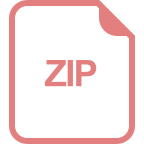
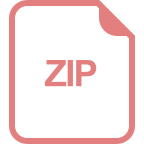