把std::map<int,int> 换成std::map<double,double> 再写一次
时间: 2023-05-23 17:06:59 浏览: 236
std::map<double, double> myMap;
myMap.insert(std::make_pair(1.0, 2.0));
myMap.insert(std::make_pair(2.5, 5.0));
myMap.insert(std::make_pair(3.1, 6.4));
for (auto it = myMap.begin(); it != myMap.end(); ++it) {
std::cout << "Key: " << it->first << " Value: " << it->second << std::endl;
}
相关问题
如何取出std::multimap<int, std::vector<std::array<double, 3> > > ret内的内容
可以使用迭代器来遍历`std::multimap<int, std::vector<std::array<double, 3> > >`类型的变量`ret`,然后将其中的元素取出来。以下是一个示例代码:
```c++
#include <iostream>
#include <map>
#include <vector>
#include <array>
int main()
{
std::multimap<int, std::vector<std::array<double, 3>>> ret = {
{1, {{1.0, 2.0, 3.0}, {4.0, 5.0, 6.0}}},
{2, {{7.0, 8.0, 9.0}, {10.0, 11.0, 12.0}}}
};
// 遍历 multimap
for(auto it = ret.begin(); it != ret.end(); ++it) {
std::cout << "key: " << it->first << std::endl;
// 遍历 vector
for(auto& vec : it->second) {
// 遍历 array
for(auto& arr : vec) {
std::cout << arr[0] << ", " << arr[1] << ", " << arr[2] << std::endl;
}
}
}
return 0;
}
```
输出结果为:
```
key: 1
1, 2, 3
4, 5, 6
key: 2
7, 8, 9
10, 11, 12
```
可以看到,在遍历`std::multimap<int, std::vector<std::array<double, 3> > >`类型的变量时,我们需要先遍历`multimap`的键值对,然后遍历对应的`vector`,最后遍历`vector`中每个`array`的元素。
std::map<std::string, std::pair<double, double>> keyValueMap存储7组数据
可以使用`std::map<std::string, std::pair<double, double>>`来存储7组数据,每组数据包括一个字符串键和两个double类型的值。以下是一个示例:
```cpp
#include <iostream>
#include <map>
int main() {
std::map<std::string, std::pair<double, double>> keyValueMap;
// 添加7组数据
keyValueMap["Data1"] = std::make_pair(1.1, 2.2);
keyValueMap["Data2"] = std::make_pair(3.3, 4.4);
keyValueMap["Data3"] = std::make_pair(5.5, 6.6);
keyValueMap["Data4"] = std::make_pair(7.7, 8.8);
keyValueMap["Data5"] = std::make_pair(9.9, 10.0);
keyValueMap["Data6"] = std::make_pair(11.1, 12.2);
keyValueMap["Data7"] = std::make_pair(13.3, 14.4);
// 遍历并打印数据
for (const auto& pair : keyValueMap) {
std::cout << "Key: " << pair.first << std::endl;
std::cout << "Value 1: " << pair.second.first << std::endl;
std::cout << "Value 2: " << pair.second.second << std::endl;
std::cout << std::endl;
}
return 0;
}
```
在上述示例中,我们创建了一个`std::map<std::string, std::pair<double, double>>`对象`keyValueMap`,并添加了7组数据。每组数据都使用`make_pair`函数创建一个包含两个double类型的值的pair,并将其与一个字符串键关联起来。然后,我们使用循环遍历了所有的键值对,并打印出键和对应的两个值。
希望这对你有帮助!
阅读全文
相关推荐
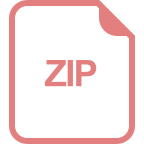













