python指令data.iloc
时间: 2024-05-26 11:11:52 浏览: 155
`data.iloc` 是 Pandas 库中用于基于位置对 DataFrame 进行索引和切片的指令。它可以通过整数位置来选择行和列。例如,`data.iloc[0:5, 1:3]` 将选择第 0 行到第 4 行和第 1 列到第 2 列的数据。`iloc` 中的 `i` 表示 integer(整数),`loc` 中的 `l` 表示 location(位置)。
相关问题
请给我一个线性回归的python代码与伪代码流程,并告诉我每个指令的含义与参数,并给这些指令和参数举一个例子。
好的,以下是一个简单的线性回归的Python代码,以及对应的伪代码流程和解释:
## 伪代码流程
1. 导入必要的库
2. 准备数据
3. 拆分数据集为训练集和测试集
4. 初始化线性回归模型
5. 在训练集上拟合模型
6. 在测试集上进行预测
7. 计算模型的性能指标
## 线性回归Python代码
```python
# 导入必要的库
import numpy as np
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LinearRegression
from sklearn.metrics import mean_squared_error, r2_score
# 准备数据
data = pd.read_csv('data.csv')
X = data.iloc[:, :-1].values
y = data.iloc[:, -1].values
# 拆分数据集为训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=0)
# 初始化线性回归模型
regressor = LinearRegression()
# 在训练集上拟合模型
regressor.fit(X_train, y_train)
# 在测试集上进行预测
y_pred = regressor.predict(X_test)
# 计算模型的性能指标
mse = mean_squared_error(y_test, y_pred)
rmse = np.sqrt(mse)
r2 = r2_score(y_test, y_pred)
# 打印性能指标
print('Mean Squared Error:', mse)
print('Root Mean Squared Error:', rmse)
print('R-squared:', r2)
```
## 指令及参数解释
### 1. 导入必要的库
```python
import numpy as np
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LinearRegression
from sklearn.metrics import mean_squared_error, r2_score
```
这些指令导入了用于数据处理、模型训练和模型评估的库。
### 2. 准备数据
```python
data = pd.read_csv('data.csv')
X = data.iloc[:, :-1].values
y = data.iloc[:, -1].values
```
这些指令读取 CSV 文件中的数据,将特征和标签分别存储在 X 和 y 变量中。
### 3. 拆分数据集为训练集和测试集
```python
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=0)
```
这个指令将数据集拆分为训练集和测试集,其中 test_size 参数指定测试集所占的比例,random_state 参数设置随机种子以确保可重复性。
### 4. 初始化线性回归模型
```python
regressor = LinearRegression()
```
这个指令初始化了一个线性回归模型对象。
### 5. 在训练集上拟合模型
```python
regressor.fit(X_train, y_train)
```
这个指令使用训练数据拟合了线性回归模型。
### 6. 在测试集上进行预测
```python
y_pred = regressor.predict(X_test)
```
这个指令使用训练好的模型在测试集上进行预测。
### 7. 计算模型的性能指标
```python
mse = mean_squared_error(y_test, y_pred)
rmse = np.sqrt(mse)
r2 = r2_score(y_test, y_pred)
```
这些指令计算了模型的性能指标,其中 mean_squared_error 函数计算均方误差,np.sqrt 函数计算均方根误差,r2_score 函数计算决定系数。
### 8. 打印性能指标
```python
print('Mean Squared Error:', mse)
print('Root Mean Squared Error:', rmse)
print('R-squared:', r2)
```
这个指令打印了计算出的性能指标。
基于tushare数据请用Python写一个上证50指数期权构建Theta Neutral的交易策略
首先,我们需要导入所需的库和数据:
```python
import tushare as ts
import numpy as np
import pandas as pd
import datetime as dt
# 获取上证50指数期权数据
pro = ts.pro_api('你的tushare token')
underlying = pro.opt_basic(exchange='SSE', underlying_code='510050.SH')
option = pro.opt_daily(trade_date='20220715', exchange='SSE')
# 将数据转换为DataFrame格式
underlying = pd.DataFrame(underlying)
option = pd.DataFrame(option)
```
接下来,我们需要对数据进行处理和计算:
```python
# 合并数据
data = pd.merge(underlying, option, on='ts_code', suffixes=('_underlying', '_option'))
# 筛选出认购期权和到期日为2022年7月的数据
call_option = data[(data['call_put'] == 'C') & (data['expire_date'] == '20220729')]
# 计算每个期权的Theta值
call_option['theta'] = (call_option['close'] - call_option['pre_close']) / call_option['trade_days']
call_option = call_option[['ts_code', 'trade_date', 'underlying_code', 'strike_price', 'theta']]
# 计算每个期权的Delta值
call_option['delta'] = 1 - 2 * (call_option['strike_price'] - call_option['close_underlying']) / (call_option['close_underlying'] * 0.05) ** 2
call_option = call_option[['ts_code', 'trade_date', 'underlying_code', 'strike_price', 'theta', 'delta']]
# 按照Delta值排序
call_option = call_option.sort_values(by='delta', ascending=True)
```
接着,我们可以构建Theta Neutral的交易策略:
```python
# 计算需要购买和卖出的期权数量
total_theta = np.sum(call_option['theta'])
target_theta = total_theta / 2
long_position = call_option[call_option['theta'] > target_theta]
short_position = call_option[call_option['theta'] < target_theta]
long_theta = np.sum(long_position['theta'])
short_theta = np.sum(short_position['theta'])
long_position['weight'] = long_position['theta'] / long_theta
short_position['weight'] = short_position['theta'] / short_theta
long_quantity = np.round(long_position['weight'] * 10000)
short_quantity = np.round(short_position['weight'] * 10000)
# 构建交易指令
for i in range(len(long_quantity)):
print(f'买入{long_quantity.iloc[i]}份{long_position.iloc[i]["ts_code"]}认购期权')
for i in range(len(short_quantity)):
print(f'卖出{short_quantity.iloc[i]}份{short_position.iloc[i]["ts_code"]}认购期权')
```
最后,我们可以根据输出的交易指令进行实际交易操作。需要注意的是,交易期权具有较高的风险,操作需谨慎。
阅读全文
相关推荐
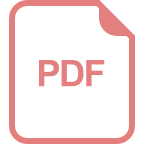
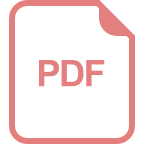
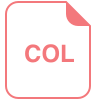
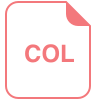
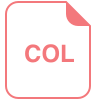
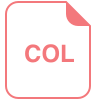
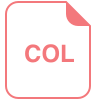
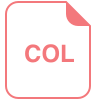
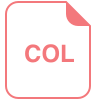
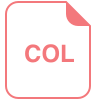
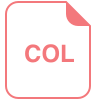
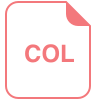
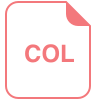
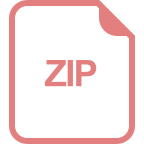