openwrt上C语言写一个简单的websocket服务器
时间: 2023-07-31 14:09:48 浏览: 152
在OpenWrt上使用C语言编写简单的WebSocket服务器,可以使用Libwebsockets库。下面是一个简单的例子:
```c
#include <libwebsockets.h>
static int callback_echo(struct lws *wsi, enum lws_callback_reasons reason, void *user, void *in, size_t len)
{
switch (reason) {
case LWS_CALLBACK_ESTABLISHED:
printf("Connection established\n");
break;
case LWS_CALLBACK_RECEIVE:
printf("Received data: %s\n", (char *)in);
// Echo back the received data
lws_write(wsi, in, len, LWS_WRITE_TEXT);
break;
default:
break;
}
return 0;
}
int main()
{
struct lws_context_creation_info info;
struct lws_context *context;
struct lws_protocols protocols[] = {
{
"echo-protocol",
callback_echo,
0,
4096,
},
{ NULL, NULL, 0, 0 } /* terminator */
};
memset(&info, 0, sizeof(info));
info.port = CONTEXT_PORT_NO_LISTEN;
info.protocols = protocols;
context = lws_create_context(&info);
if (!context) {
printf("Failed to create LWS context\n");
return -1;
}
while (1) {
lws_service(context, 50);
}
lws_context_destroy(context);
return 0;
}
```
这个例子创建了一个名为“echo-protocol”的协议,当客户端连接时,会打印一条消息。当接收到数据时,会将其回显回客户端。
注意:这个例子不包含任何安全性和错误处理,仅用于演示目的。在实际生产环境中,需要使用SSL加密连接,并进行适当的错误处理和安全性保护。
阅读全文
相关推荐
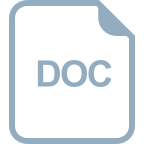
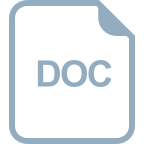
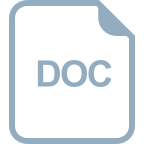




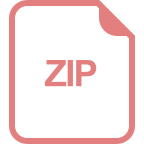
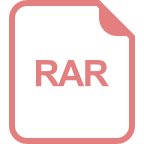
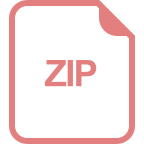
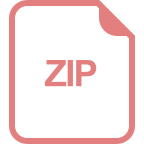




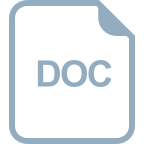
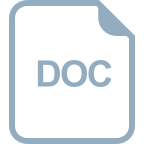
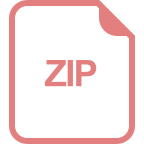