地图着色问题java
时间: 2023-10-11 14:24:32 浏览: 201
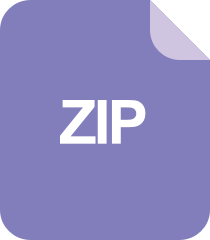
地图着色问题
地图着色问题是一种经典的图论问题,它的主要目标是为地图上的每一个区域分配一种颜色,使得相邻的区域颜色不同。该问题可以使用Java语言进行解决。
以下是地图着色问题的Java实现示例:
```java
import java.util.*;
public class MapColoringProblem {
static int V = 4; // 定义图的顶点数
static int color[]; // 定义每个顶点的颜色
// 检查当前顶点v是否可以着色为c
static boolean isSafe(int v, int graph[][], int color[], int c) {
for (int i = 0; i < V; i++)
if (graph[v][i] == 1 && c == color[i])
return false;
return true;
}
// 递归函数,为每个顶点着色
static boolean graphColoringUtil(int graph[][], int m, int color[], int v) {
if (v == V)
return true;
for (int c = 1; c <= m; c++) {
if (isSafe(v, graph, color, c)) {
color[v] = c;
if (graphColoringUtil(graph, m, color, v + 1) == true)
return true;
color[v] = 0;
}
}
return false;
}
// 主函数,解决地图着色问题
static boolean graphColoring(int graph[][], int m) {
color = new int[V];
for (int i = 0; i < V; i++)
color[i] = 0;
if (graphColoringUtil(graph, m, color, 0) == false) {
System.out.println("无法找到合法的着色方案!");
return false;
}
System.out.println("找到的合法着色方案为:");
for (int i = 0; i < V; i++)
System.out.print(color[i] + " ");
System.out.println();
return true;
}
public static void main(String args[]) {
int graph[][] = {{0, 1, 1, 1},
{1, 0, 1, 0},
{1, 1, 0, 1},
{1, 0, 1, 0}};
int m = 3; // 每个顶点可以使用的颜色数
graphColoring(graph, m);
}
}
```
在上述示例中,我们首先定义了一个包含4个顶点的图(即4个区域的地图),然后指定每个顶点可以使用的颜色数为3。接着我们调用`graphColoring`函数来解决地图着色问题,该函数首先初始化每个顶点的颜色为0,然后逐一为每个顶点着色,直到所有顶点都被着色。在递归过程中,我们使用`isSafe`函数来检查当前顶点是否可以着色为指定的颜色。如果找到了合法的着色方案,我们就输出每个顶点的颜色。如果找不到合法的着色方案,我们就输出相应的提示信息。
阅读全文
相关推荐
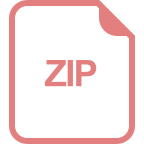


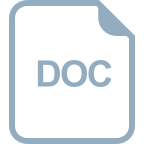
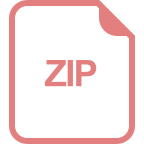
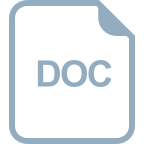
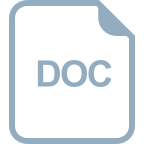

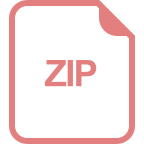
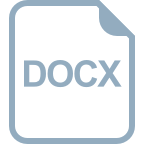
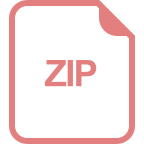
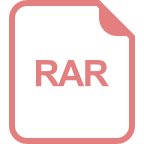
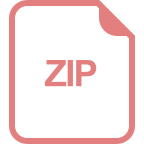
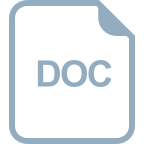
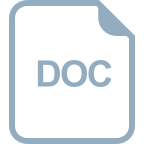
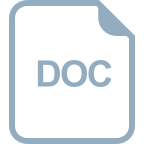
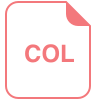