本关任务:接收给定的一行字符串(某学生各科成绩),当某科成绩大于 100 或者小于 0 时,抛出一个异常(Exception),提示“成绩录入异常”。最后统计该学生各科平均成绩。java
时间: 2024-12-13 12:27:09 浏览: 7
在 Java 中,你可以按照下面的方式来完成这个任务:
首先,你需要创建一个 `Student` 类,包含各个学科的成绩作为属性,并提供一个计算平均成绩的方法。为了处理成绩异常,可以使用 try-catch-finally 语句块。
```java
public class Student {
private double[] scores; // 学生各科成绩
public Student(double[] scores) {
this.scores = scores;
}
// 检查成绩并计算平均分
public double calculateAverage() throws Exception {
if (scores == null || scores.length < 1) {
throw new IllegalArgumentException("Invalid score array");
}
double sum = 0;
for (double score : scores) {
if (score < 0 || score > 100) {
throw new Exception("Score out of range: " + score);
}
sum += score;
}
return sum / scores.length;
}
}
```
然后,在主程序中,你可以这样做:
```java
public static void main(String[] args) {
String input = "85,90,110,75"; // 示例输入,每个数字之间用逗号分隔
String[] scoresStr = input.split(",");
double[] scores = new double[scoresStr.length];
try {
for (int i = 0; i < scores.length; i++) {
scores[i] = Double.parseDouble(scoresStr[i]);
}
Student student = new Student(scores);
double average = student.calculateAverage();
System.out.println("Average score: " + average);
} catch (NumberFormatException e) {
System.err.println("Invalid score format, please enter numbers only.");
} catch (Exception e) {
System.err.println(e.getMessage());
} finally {
// 在这里添加清理操作或其他必要代码
}
}
```
在这个例子中,如果输入包含非数字字符,会捕获 `NumberFormatException`;如果成绩超出范围,会抛出我们自定义的异常。最后,如果没有异常,将打印学生的平均成绩。如果你需要,可以在 `finally` 区段中加入一些清理工作,比如关闭文件流、数据库连接等。
阅读全文
相关推荐
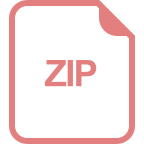
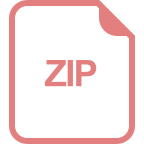
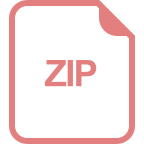
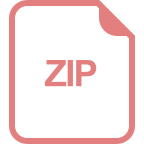
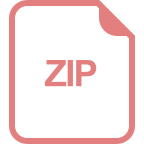
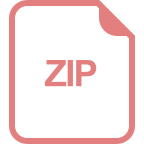
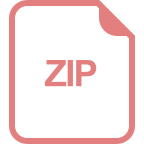
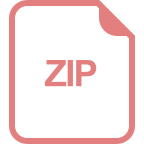
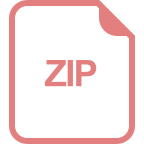
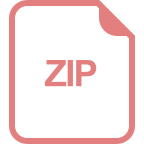
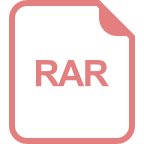
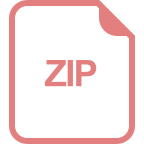
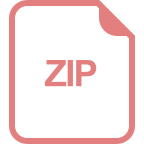
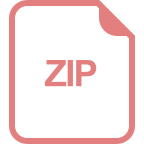
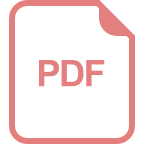