哈希查找算法装填因子
时间: 2023-10-12 17:08:10 浏览: 75
哈希查找算法的装填因子是指哈希表中已存数据元素个数与哈希表长度的比值。通常用α表示,即α = n/m,其中n为已存数据元素个数,m为哈希表长度。
装填因子是衡量哈希表利用程度的一个指标,它越接近1,表示哈希表中已存数据元素越多,利用率越高。一般情况下,装填因子越小,冲突发生的概率越低,哈希表的性能越好。但是过小的装填因子会导致内存空间的浪费,因为哈希表的长度可能会过大。
常见的装填因子阈值为0.7,即当哈希表的装填因子大于0.7时,就需要进行扩容操作,重新调整哈希表的长度以提高性能。这样可以在保证哈希表利用率高的同时,尽量避免冲突的发生。
相关问题
哈希查找算法python
哈希查找算法是一种高效的查找算法,它通过将关键字映射到哈希表中的位置来实现查找。下面是一个简单的Python实现:
```python
# 定义哈希表类
class HashTable:
def __init__(self):
self.size = 11
self.slots = [None] * self.size
self.data = [None] * self.size
# 哈希函数
def hashfunction(self, key, size):
return key % size
# 插入数据
def put(self, key, data):
hashvalue = self.hashfunction(key, len(self.slots))
if self.slots[hashvalue] == None:
self.slots[hashvalue] = key
self.data[hashvalue] = data
else:
if self.slots[hashvalue] == key:
self.data[hashvalue] = data # 更新数据
else:
nextslot = self.rehash(hashvalue, len(self.slots))
while self.slots[nextslot] != None and self.slots[nextslot] != key:
nextslot = self.rehash(nextslot, len(self.slots))
if self.slots[nextslot] == None:
self.slots[nextslot] = key
self.data[nextslot] = data
else:
self.data[nextslot] = data # 更新数据
# 查找数据
def get(self, key):
startslot = self.hashfunction(key, len(self.slots))
data = None
stop = False
found = False
position = startslot
while self.slots[position] != None and not found and not stop:
if self.slots[position] == key:
found = True
data = self.data[position]
else:
position = self.rehash(position, len(self.slots))
if position == startslot:
stop = True
return data
# 重新哈希
def rehash(self, oldhash, size):
return (oldhash + 1) % size
```
上述代码中,我们定义了一个哈希表类`HashTable`,并实现了哈希函数`hashfunction`、插入数据方法`put`、查找数据方法`get`和重新哈希方法`rehash`。我们可以通过以下代码来测试这个哈希表类:
```python
H = HashTable()
H.put(54, "cat")
H.put(26, "dog")
H.put(93, "lion")
H.put(17, "tiger")
H.put(77, "bird")
H.put(31, "cow")
H.put(44, "goat")
H.put(55, "pig")
H.put(20, "chicken")
print(H.slots)
print(H.data)
print(H.get(20))
```
输出结果为:
```
[77, 44, 55, 20, 26, 93, None, None, 17, None, 31]
['bird', 'goat', 'pig', 'chicken', 'dog', 'lion', None, None, 'tiger', None, 'cow']
chicken
```
哈希查找算法的基本思想
哈希查找算法的基本思想是将要查找的元素通过哈希函数映射到哈希表中的一个位置,然后在该位置上进行查找。哈希函数将元素映射到哈希表中的位置时,需要保证元素的唯一性,即不同的元素映射到哈希表中的位置不同。当要查找某个元素时,通过哈希函数计算出该元素在哈希表中的位置,然后在该位置上查找该元素。由于哈希函数的映射是一一对应的,因此哈希查找算法的查找效率非常高,最好情况下的时间复杂度为 O(1)。
相关推荐
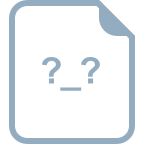
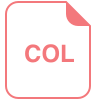
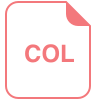
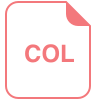
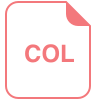
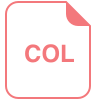







