用C语言写一个哈希查找算法
时间: 2023-12-29 12:56:10 浏览: 86
以下是一个简单的哈希查找算法的C语言实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_SIZE 100 // 哈希表的最大长度
typedef struct {
char key[20]; // 关键字
int value; // 值
} HashNode;
void initHashTable(HashNode* hashTable[]) {
for (int i = 0; i < MAX_SIZE; i++) {
hashTable[i] = NULL; // 初始化为NULL
}
}
int hash(char* key) {
int hashValue = 0;
for (int i = 0; i < strlen(key); i++) {
hashValue = (hashValue * 31 + key[i]) % MAX_SIZE; // 哈希函数
}
return hashValue;
}
void insert(HashNode* hashTable[], char* key, int value) {
int hashValue = hash(key);
HashNode* node = (HashNode*)malloc(sizeof(HashNode));
strcpy(node->key, key);
node->value = value;
hashTable[hashValue] = node; // 直接插入哈希表
}
int search(HashNode* hashTable[], char* key) {
int hashValue = hash(key);
if (hashTable[hashValue] != NULL && strcmp(hashTable[hashValue]->key, key) == 0) {
return hashTable[hashValue]->value; // 查找成功,返回对应的值
} else {
return -1; // 查找失败,返回-1
}
}
int main() {
HashNode* hashTable[MAX_SIZE];
initHashTable(hashTable);
insert(hashTable, "apple", 100);
insert(hashTable, "banana", 200);
insert(hashTable, "cherry", 300);
printf("%d\n", search(hashTable, "apple")); // 输出100
printf("%d\n", search(hashTable, "banana")); // 输出200
printf("%d\n", search(hashTable, "cherry")); // 输出300
printf("%d\n", search(hashTable, "orange")); // 输出-1
return 0;
}
```
这是一个简单的哈希查找算法,具体实现可以根据具体需求进行修改。
阅读全文
相关推荐

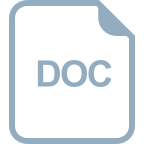

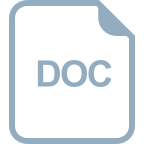
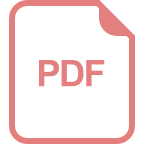
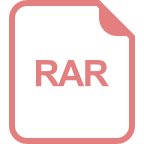
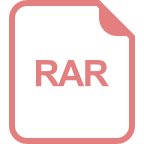
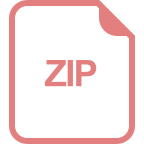
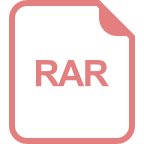
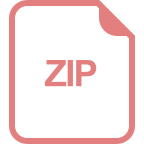
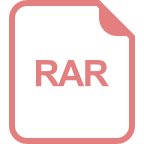
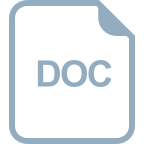
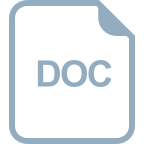
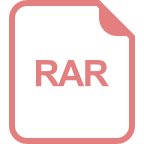