private Integer countMobileNum(AnalysisInfoVO graphAttrInfo, Integer mobileNumTotal) { String mobileNo1 = graphAttrInfo.getMobileNo1(); String mobileNo2 = graphAttrInfo.getMobileNo2(); String mobileNo3 = graphAttrInfo.getMobileNo3(); if (StringUtils.isNotEmpty(mobileNo1)){ mobileNumTotal= mobileNumTotal + 1; } if (StringUtils.isNotEmpty(mobileNo2)){ mobileNumTotal= mobileNumTotal + 1; } if (StringUtils.isNotEmpty(mobileNo3)){ mobileNumTotal= mobileNumTotal + 1; } return mobileNumTotal; } 帮我优化这段代码
时间: 2024-01-16 12:05:11 浏览: 34
可以使用循环来遍历 `mobileNo` 字段,避免重复代码的出现。同时,将 `StringUtils.isNotEmpty()` 方法替换为直接判断字符串是否为 `null` 或者长度是否为 0 的方式,可以提高代码可读性和执行效率。优化后的代码如下所示:
```
private Integer countMobileNum(AnalysisInfoVO graphAttrInfo, Integer mobileNumTotal) {
for (int i = 1; i <= 3; i++) {
String mobileNo = graphAttrInfo.getMobileNo(i);
if (mobileNo != null && mobileNo.length() > 0) {
mobileNumTotal++;
}
}
return mobileNumTotal;
}
```
在优化后的代码中,我们使用了一个循环来遍历 `mobileNo` 字段,从 1 到 3 分别获取对应的字段值。使用 `mobileNo != null && mobileNo.length() > 0` 来判断字符串是否为空或者长度是否为 0,如果满足条件,就将 `mobileNumTotal` 加一。最后返回 `mobileNumTotal` 的值即可。
相关问题
Out of range value for column 'MobileNo' at row 1
这个错误提示通常是由于在向MySQL数据库插入数据时,MobileNo字段的值超出了该字段所能存储的范围。可能是因为MobileNo字段定义为了过小的整数类型或者字符类型。
解决方法可以是:
1. 扩大MobileNo字段的类型,例如将int类型扩大到bigint类型,或者将varchar类型扩大到更大的长度。
2. 检查插入的数据是否符合MobileNo字段的定义范围。可以通过查询MobileNo字段的类型和长度来确定其可存储的最大值。
3. 确认插入的数据是否存在错误。例如,如果MobileNo字段定义为整数类型,则插入的数据必须是整数,如果插入的是字符串,则会出现该错误。
4. 如果以上方法无法解决问题,则可能需要修改表结构或者重新设计数据模型,以适应MobileNo字段的需求。
优化这段代码: String modeMsg = MsgModelEnum.getModeMsg(msgCode); if(StringUtils.isEmpty(modeMsg)){ return null; } String msg = String.format(modeMsg, verifyCode); Map<String,Object> body = new HashMap<>(16); body.put("BAWELX","740000"); body.put("CHSHDM","7400"); body.put(qudaoo,"74"); body.put("JIGOCD","0001"); body.put("JIGOBS","1"); body.put("TELNO0",mobileNo); body.put("NEIRNG",msg); String sendJsonParse = JsonParse.sendJsonParse(body, selfSysName, msgPlate, cnsmrSvrNode, ESBInterfaceEnum.SEND_MSG_CODE.getSvcCd(), ESBInterfaceEnum.SEND_MSG_CODE.getScnCd(),msgSplrchnltp,yytxTxnchnltp,null); String acceptData = sendSocket(sendJsonParse, msgPlate, null); JSONObject resultJson = JSONObject.parseObject(acceptData); JSONObject sysHead = resultJson.getJSONObject(sysHeadDetail); if(aaaaaaa.equals(sysHead.getString(retCd))){ return new SuccessTip<String>(); }else{ String retMsg = sysHead.getString("RetMsg"); return ErrorTip.defaultErrorTip(retMsg); }
可以优化的地方如下:
1. 使用 `StringUtils.isBlank()` 方法代替 `StringUtils.isEmpty()` 方法和 `String` 类的 `trim()` 方法,以判断字符串是否为空或者全部由空白字符组成。
2. 可以将 `msgPlate`、`cnsmrSvrNode`、`msgSplrchnltp`、`yytxTxnchnltp` 这些字符串参数抽取出来,定义为常量或者枚举类型,提高代码的可维护性。
3. 使用 `LinkedHashMap` 代替 `HashMap`,以确保添加元素的顺序与读取元素的顺序一致,提高代码的可读性。
4. 将返回类型改为具体的类型,避免使用通用类型 `Object`,提高代码的可读性。
综上所述,优化后的代码如下:
```
String modeMsg = MsgModelEnum.getModeMsg(msgCode);
if(StringUtils.isBlank(modeMsg)){
return null;
}
String msg = String.format(modeMsg, verifyCode);
Map<String,Object> body = new LinkedHashMap<>();
body.put("BAWELX", "740000");
body.put("CHSHDM", "7400");
body.put(qudaoo, "74");
body.put("JIGOCD", "0001");
body.put("JIGOBS", "1");
body.put("TELNO0", mobileNo);
body.put("NEIRNG", msg);
String sendJsonParse = JsonParse.sendJsonParse(body, selfSysName, msgPlate, cnsmrSvrNode,
ESBInterfaceEnum.SEND_MSG_CODE.getSvcCd(), ESBInterfaceEnum.SEND_MSG_CODE.getScnCd(),
msgSplrchnltp, yytxTxnchnltp, null);
String acceptData = sendSocket(sendJsonParse, msgPlate, null);
JSONObject resultJson = JSONObject.parseObject(acceptData);
JSONObject sysHead = resultJson.getJSONObject(sysHeadDetail);
if(aaaaaaa.equals(sysHead.getString(retCd))){
return new SuccessTip<>("Success");
}else{
String retMsg = sysHead.getString("RetMsg");
return new ErrorTip<>(retMsg);
}
```
相关推荐
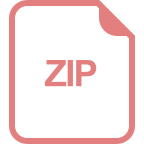
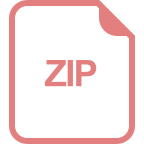
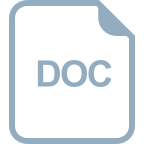




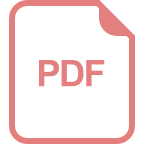
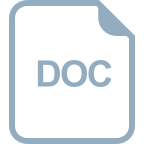
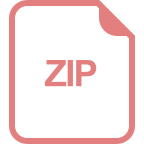
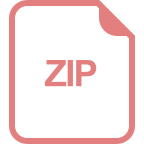
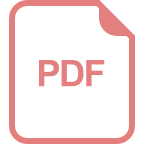
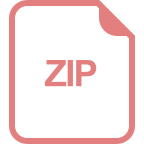
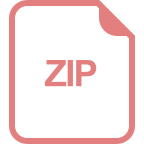
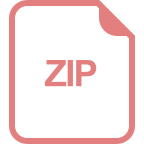