在windows 下用unity添加蓝牙搜索功能需要什么第三方库
时间: 2023-12-14 18:39:04 浏览: 42
在 Windows 下使用 Unity 添加蓝牙搜索功能,需要使用 Windows.Devices.Bluetooth 命名空间提供的 API。不需要额外安装第三方库。
以下是使用 Windows.Devices.Bluetooth API 搜索蓝牙设备的示例代码:
```csharp
using UnityEngine;
using System.Collections.Generic;
using System.Threading.Tasks;
using Windows.Devices.Enumeration;
using Windows.Devices.Bluetooth;
using Windows.Devices.Bluetooth.Advertisement;
public class BluetoothScanner : MonoBehaviour
{
private BluetoothLEAdvertisementWatcher watcher;
private List<BluetoothDevice> devices = new List<BluetoothDevice>();
private async void Start()
{
// 初始化 watcher
watcher = new BluetoothLEAdvertisementWatcher();
watcher.Received += Watcher_Received;
// 开始搜索
watcher.Start();
// 等待搜索完成
await Task.Delay(5000);
// 停止搜索
watcher.Stop();
}
private void Watcher_Received(BluetoothLEAdvertisementWatcher sender, BluetoothLEAdvertisementReceivedEventArgs args)
{
// 处理搜索到的设备
BluetoothDevice device = new BluetoothDevice(args.BluetoothAddress, args.RawSignalStrengthInDBm);
if (!devices.Contains(device))
{
devices.Add(device);
Debug.Log("Found device: " + device.Name);
}
}
private void OnDestroy()
{
// 释放 watcher
if (watcher != null)
{
watcher.Stop();
watcher.Received -= Watcher_Received;
watcher = null;
}
}
}
public class BluetoothDevice
{
public ulong Address { get; private set; }
public string Name { get; private set; }
public short SignalStrength { get; private set; }
public BluetoothDevice(ulong address, short signalStrength)
{
Address = address;
SignalStrength = signalStrength;
Name = "Unknown";
}
public override bool Equals(object obj)
{
if (obj is BluetoothDevice other)
{
return Address == other.Address;
}
return false;
}
public override int GetHashCode()
{
return Address.GetHashCode();
}
}
```
在代码中,我们创建了一个 BluetoothLEAdvertisementWatcher 对象,并在 Start() 方法中调用 Start() 方法开始搜索。当搜索到设备时,Watcher_Received() 方法会被调用并处理搜索到的设备。最后,在 OnDestroy() 方法中调用 Stop() 方法停止搜索。
需要注意的是,使用 Windows.Devices.Bluetooth API 搜索蓝牙设备需要在 Unity 中使用 .NET 4.x API,而不是 Unity 默认的 .NET 3.5 API。可以在 Unity 编辑器中选择 Edit -> Project Settings -> Player -> Other Settings,然后将 Scripting Runtime Version 设置为 .NET 4.x Equivalent。
相关推荐
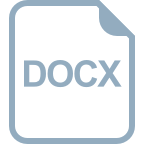
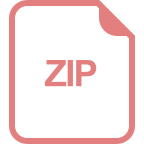
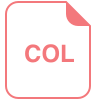
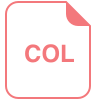
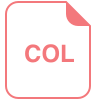
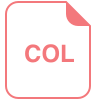
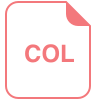








