现有数据文件‘result.csv',包含CustomerID,RS,FS,MS,Label和CustomerType这几个字段,请你基于django框架为这个文件设计一个网页,要求能实现对该文件的分页展示,并能通过CustomerID进行单条数据查询,使用 Bootstrap 和 DataTables 插件
时间: 2023-12-12 09:03:30 浏览: 33
好的,我可以为您提供一些设计思路。首先,您需要安装 Django 和 DataTables 插件。
1. 创建 Django 项目和应用程序
使用 Django 命令行工具创建项目和应用程序:
```
django-admin startproject myproject
cd myproject
python manage.py startapp myapp
```
2. 创建模型
在 models.py 文件中定义模型:
```python
from django.db import models
class Customer(models.Model):
customer_id = models.IntegerField(primary_key=True)
rs = models.IntegerField()
fs = models.IntegerField()
ms = models.IntegerField()
label = models.CharField(max_length=20)
customer_type = models.CharField(max_length=20)
```
3. 创建视图和模板
在 views.py 文件中定义视图:
```python
from django.shortcuts import render
from django.core.paginator import Paginator
from myapp.models import Customer
def customer_list(request):
customer_list = Customer.objects.all()
paginator = Paginator(customer_list, 10) # 每页显示10条记录
page = request.GET.get('page')
customers = paginator.get_page(page)
return render(request, 'customer_list.html', {'customers': customers})
def customer_detail(request, customer_id):
customer = Customer.objects.get(pk=customer_id)
return render(request, 'customer_detail.html', {'customer': customer})
```
在 urls.py 文件中定义 URL:
```python
from django.urls import path
from myapp.views import customer_list, customer_detail
urlpatterns = [
path('', customer_list, name='customer_list'),
path('<int:customer_id>/', customer_detail, name='customer_detail'),
]
```
在 customer_list.html 模板中使用 DataTables 插件渲染表格:
```html
{% extends 'base.html' %}
{% block content %}
<h1>Customer List</h1>
<table id="customer-table" class="table table-striped table-bordered">
<thead>
<tr>
<th>Customer ID</th>
<th>RS</th>
<th>FS</th>
<th>MS</th>
<th>Label</th>
<th>Customer Type</th>
</tr>
</thead>
<tbody>
{% for customer in customers %}
<tr>
<td><a href="{% url 'customer_detail' customer.customer_id %}">{{ customer.customer_id }}</a></td>
<td>{{ customer.rs }}</td>
<td>{{ customer.fs }}</td>
<td>{{ customer.ms }}</td>
<td>{{ customer.label }}</td>
<td>{{ customer.customer_type }}</td>
</tr>
{% endfor %}
</tbody>
</table>
{% if customers.has_other_pages %}
<ul class="pagination">
{% if customers.has_previous %}
<li class="page-item"><a class="page-link" href="?page={{ customers.previous_page_number }}">«</a></li>
{% endif %}
{% for i in customers.paginator.page_range %}
{% if customers.number == i %}
<li class="page-item active"><a class="page-link" href="#">{{ i }}</a></li>
{% else %}
<li class="page-item"><a class="page-link" href="?page={{ i }}">{{ i }}</a></li>
{% endif %}
{% endfor %}
{% if customers.has_next %}
<li class="page-item"><a class="page-link" href="?page={{ customers.next_page_number }}">»</a></li>
{% endif %}
</ul>
{% endif %}
<script>
$(document).ready(function() {
$('#customer-table').DataTable();
});
</script>
{% endblock %}
```
在 customer_detail.html 模板中显示单条数据:
```html
{% extends 'base.html' %}
{% block content %}
<h1>Customer Detail</h1>
<dl class="row">
<dt class="col-sm-3">Customer ID</dt>
<dd class="col-sm-9">{{ customer.customer_id }}</dd>
<dt class="col-sm-3">RS</dt>
<dd class="col-sm-9">{{ customer.rs }}</dd>
<dt class="col-sm-3">FS</dt>
<dd class="col-sm-9">{{ customer.fs }}</dd>
<dt class="col-sm-3">MS</dt>
<dd class="col-sm-9">{{ customer.ms }}</dd>
<dt class="col-sm-3">Label</dt>
<dd class="col-sm-9">{{ customer.label }}</dd>
<dt class="col-sm-3">Customer Type</dt>
<dd class="col-sm-9">{{ customer.customer_type }}</dd>
</dl>
{% endblock %}
```
4. 配置 Bootstrap 和 DataTables
将 Bootstrap 和 DataTables 的 CSS 和 JavaScript 文件复制到静态文件目录中,并在 base.html 模板中引入:
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>{% block title %}My Project{% endblock %}</title>
<link rel="stylesheet" href="https://cdn.bootcdn.net/ajax/libs/twitter-bootstrap/4.5.2/css/bootstrap.min.css">
<link rel="stylesheet" href="https://cdn.datatables.net/1.10.22/css/dataTables.bootstrap4.min.css">
</head>
<body>
<nav class="navbar navbar-expand-lg navbar-light bg-light">
<a class="navbar-brand" href="#">My Project</a>
<button class="navbar-toggler" type="button" data-toggle="collapse" data-target="#navbarNav" aria-controls="navbarNav" aria-expanded="false" aria-label="Toggle navigation">
<span class="navbar-toggler-icon"></span>
</button>
<div class="collapse navbar-collapse" id="navbarNav">
<ul class="navbar-nav">
<li class="nav-item">
<a class="nav-link" href="{% url 'customer_list' %}">Customer List</a>
</li>
</ul>
</div>
</nav>
<div class="container">
{% block content %}{% endblock %}
</div>
<script src="https://cdn.bootcdn.net/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
<script src="https://cdn.bootcdn.net/ajax/libs/twitter-bootstrap/4.5.2/js/bootstrap.min.js"></script>
<script src="https://cdn.datatables.net/1.10.22/js/jquery.dataTables.min.js"></script>
<script src="https://cdn.datatables.net/1.10.22/js/dataTables.bootstrap4.min.js"></script>
</body>
</html>
```
5. 迁移数据库
运行以下命令迁移数据库:
```
python manage.py makemigrations
python manage.py migrate
```
6. 导入数据
将 result.csv 文件导入到数据库中:
```
python manage.py shell
>>> from myapp.models import Customer
>>> import csv
>>> with open('result.csv') as f:
... reader = csv.reader(f)
... for row in reader:
... customer = Customer(customer_id=row[0], rs=row[1], fs=row[2], ms=row[3], label=row[4], customer_type=row[5])
... customer.save()
```
7. 启动服务
运行以下命令启动服务:
```
python manage.py runserver
```
现在,您可以在浏览器中访问 http://localhost:8000/ 查看网页,通过 http://localhost:8000/<customer_id>/ 进行单条数据查询。
相关推荐
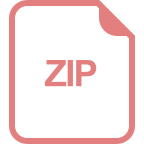
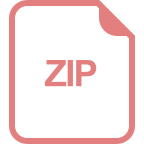
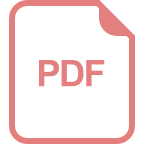














