使用spring boot写图片的上传下载
时间: 2024-01-08 07:04:15 浏览: 77
要使用Spring Boot来实现图片的上传下载,可以使用Spring Boot提供的Multipart文件上传功能和Resource资源访问功能。
首先,在pom.xml文件中添加以下依赖:
```
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-webflux</artifactId>
</dependency>
```
然后,在application.properties文件中添加以下配置:
```
# 储存位置
upload.dir=/path/to/upload/dir
```
接下来,创建一个Controller类来处理上传和下载请求:
```
import org.springframework.core.io.InputStreamResource;
import org.springframework.core.io.Resource;
import org.springframework.http.MediaType;
import org.springframework.http.ResponseEntity;
import org.springframework.stereotype.Controller;
import org.springframework.util.StringUtils;
import org.springframework.web.bind.annotation.*;
import org.springframework.web.multipart.MultipartFile;
import java.io.*;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
@Controller
public class FileController {
private static final String UPLOAD_DIR = "/path/to/upload/dir";
@PostMapping("/upload")
public ResponseEntity<String> handleFileUpload(@RequestParam("file") MultipartFile file) {
String filename = StringUtils.cleanPath(file.getOriginalFilename());
try {
if (file.isEmpty()) {
throw new RuntimeException("文件为空");
}
if (filename.contains("..")) {
throw new RuntimeException("无效的文件名");
}
try (InputStream inputStream = file.getInputStream()) {
Files.copy(inputStream, Paths.get(UPLOAD_DIR).resolve(filename));
}
} catch (IOException e) {
throw new RuntimeException("上传失败");
}
return ResponseEntity.ok("上传成功");
}
@GetMapping("/download")
public ResponseEntity<Resource> serveFile(@RequestParam("filename") String filename) {
Path file = Paths.get(UPLOAD_DIR).resolve(filename);
Resource resource = null;
try {
resource = new InputStreamResource(Files.newInputStream(file));
} catch (IOException e) {
throw new RuntimeException("文件不存在");
}
return ResponseEntity.ok()
.contentLength(file.toFile().length())
.contentType(MediaType.parseMediaType("application/octet-stream"))
.body(resource);
}
}
```
在上面的Controller类中,我们使用了MultipartFile来接收上传的文件,并使用Files.copy方法将文件复制到指定的目录下。在下载文件时,我们使用了Resource和InputStreamResource来读取文件内容,并将其包装成ResponseEntity对象返回给客户端。
最后,启动应用程序,可以使用以下命令上传文件:
```
curl -F "file=@/path/to/file.jpg" http://localhost:8080/upload
```
也可以使用以下命令下载文件:
```
curl -OJ http://localhost:8080/download?filename=file.jpg
```
其中,-O选项表示将文件保存到本地,-J选项表示使用文件名作为保存文件的名称。
阅读全文
相关推荐
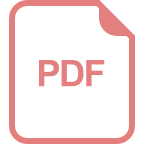
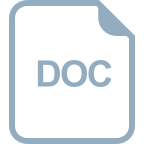
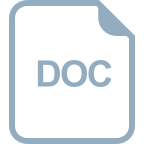
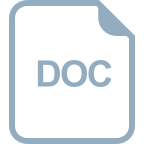
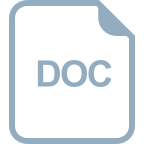
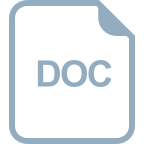
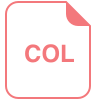








