用c语言和二分查找解决这个问题描述:描述:Given an array, find the number of [i,j] (i and j are the indexes of the array (数组下标), not values) tuples in the array that satisfy array[i]+array[j]<=k. 输入:There are several test cases. For each test case, The first line contains two positive integers n (1<=n<=10^5) and k (1<=k<=10^18). The second line contains n integers, and the ith integer represents the value of array[i](1<= array[i]<=10^9). 输出:For each test case, output an integer representing the answer.
时间: 2024-02-18 11:05:11 浏览: 36
好的,让我解释一下这个问题的意思。题目要求我们在一个给定的数组中,找到所有满足条件 array[i]+array[j]<=k 的下标对 [i,j],并输出满足条件的下标对数量。
下面是使用 C 语言和二分查找算法的解法:
```c
#include<stdio.h>
#include<stdlib.h>
#define MAXN 100005
long long a[MAXN];
int cmp(const void *a, const void *b)
{
return *(long long *)a - *(long long *)b;
}
int binary_search(long long *a, int l, int r, long long x)
{
int mid;
while (l <= r) {
mid = l + (r - l) / 2;
if (a[mid] <= x) {
l = mid + 1;
} else {
r = mid - 1;
}
}
return r;
}
int main()
{
int n;
long long k;
while (scanf("%d%lld", &n, &k) != EOF) {
for (int i = 0; i < n; i++) {
scanf("%lld", &a[i]);
}
qsort(a, n, sizeof(long long), cmp);
long long ans = 0;
for (int i = 0; i < n; i++) {
int j = binary_search(a, i+1, n-1, k-a[i]);
ans += j - i;
}
printf("%lld\n", ans);
}
return 0;
}
```
首先,我们需要对输入的数组进行排序,这样可以方便我们使用二分查找算法。然后,我们遍历数组中的每个元素 a[i],在剩下的 a[i+1] 至 a[n-1] 中寻找满足条件的元素 a[j],即 a[j] <= k - a[i]。我们可以使用二分查找算法在剩下的元素中查找满足条件的元素,从而求得满足条件的下标对数量。最后,我们将所有满足条件的数量累加起来,即为最终的答案。
注意,题目中给出的数组下标从 0 开始,而二分查找算法中的下标 i、j 都从 1 开始,因此在二分查找函数中,我们需要将 i+1 转换为实际的下标。
时间复杂度为 O(nlogn)。
相关推荐
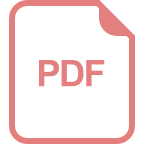
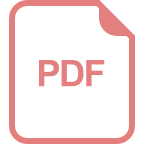














