用c语言和贪心算法解决这个问题:描述 Given an integer V representing the total amount of money you need to change, supposing you have an infinite supply of { 1, 2, 5, 10, 20, 50, 100} valued coins, what is the minimum number of coins needed to make the change? 输入 The first line includes an integer T (T<50), the number of test cases. Every test case is an integer V (1<=V<=100000). 输出 For each test case, output the minimum number of coins that you need to make the change.
时间: 2024-02-20 20:57:22 浏览: 20
好的,这是一个很典型的贪心算法问题。我们可以按照从大到小的顺序依次选择硬币,直到总金额达到所需的金额。以下是代码实现:
```c
#include<stdio.h>
int main(){
int T, V, coins[7] = {100, 50, 20, 10, 5, 2, 1};
scanf("%d", &T);
while(T--){
scanf("%d", &V);
int ans = 0;
for(int i = 0; i < 7; i++){
while(V >= coins[i]){
V -= coins[i];
ans++;
}
}
printf("%d\n", ans);
}
return 0;
}
```
首先输入测试用例的数量 T,然后依次输入每个测试用例中所需的金额 V。接着用一个数组 coins 存储硬币的面值,按照从大到小的顺序排列。接下来,我们用一个循环依次选择硬币,直到总金额达到所需的金额。最后输出所需的硬币数量即可。
相关问题
描述 Given an integer V representing the total amount of money you need to change, supposing you have an infinite supply of { 1, 2, 5, 10, 20, 50, 100} valued coins, what is the minimum number of coins needed to make the change? 输入 The first line includes an integer T (T<50), the number of test cases. Every test case is an integer V (1<=V<=100000). 输出 For each test case, output the minimum number of coins that you need to make the change.
这是一个典型的动态规划问题。我们可以定义一个长度为 V+1 的数组 dp,其中 dp[i] 表示凑出 i 元所需的最小硬币数。对于每一个硬币面值,我们可以枚举它凑出的所有金额,从而更新 dp 数组。具体实现如下:
```python
coin_values = [1, 2, 5, 10, 20, 50, 100]
max_value = max(coin_values)
dp = [float('inf')] * (max_value + 1)
dp[0] = 0
# 预处理出所有可能的金额
for i in range(1, max_value + 1):
for coin in coin_values:
if i >= coin:
dp[i] = min(dp[i], dp[i - coin] + 1)
# 处理每个测试用例
t = int(input())
for _ in range(t):
v = int(input())
ans = 0
for i in range(0, v + 1, max_value):
# 对于每个区间,使用预处理出的 dp 数组求解最小硬币数
ans += dp[min(v - i, max_value)]
print(ans)
```
代码中的 dp 数组预处理出了所有可能的金额,最大值为 coin_values 中最大的硬币面值。在处理每个测试用例时,我们将金额拆分成若干个区间,每个区间长度为 max_value,使用预处理出的 dp 数组求解区间内的最小硬币数,最后将所有区间内的硬币数相加即可得到答案。
用c语言和贪心算法解决这个问题:描述 Given N TV programs with each having a begin time and an end time, compute how many TV programs you can watch at most. 输入 There are multiple test cases. The first line of each test case contains an integer N (1<=N<=1000), the number of TV programs. Then N lines follow, with every line containing two integers Bi and Ei (1<=Bi<Ei<=INT_MAX), representing the begin time and end time of TV program i. The inputs terminate at the end of the file (EOF). 输出 For each test case, output a line containing an integer which is the number of TV programs that you can watch at most.
好的,我会为您解答这个问题。首先,这是一个经典的贪心算法问题,我们可以按照结束时间从早到晚对所有电视节目进行排序,然后依次选择结束时间最早的电视节目,并将其结束时间作为下一个选择的起始时间,直到所有电视节目都被选择完。下面是用C语言实现的代码:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct {
int start;
int end;
} Program;
int cmp(const void* a, const void* b) {
Program* p1 = (Program*)a;
Program* p2 = (Program*)b;
return p1->end - p2->end;
}
int main() {
int n;
while (scanf("%d", &n) != EOF) {
Program* programs = (Program*)malloc(n * sizeof(Program));
for (int i = 0; i < n; i++) {
scanf("%d%d", &programs[i].start, &programs[i].end);
}
qsort(programs, n, sizeof(Program), cmp);
int count = 0, end = 0;
for (int i = 0; i < n; i++) {
if (programs[i].start >= end) {
count++;
end = programs[i].end;
}
}
printf("%d\n", count);
free(programs);
}
return 0;
}
```
代码中,我们定义了一个结构体 `Program` 来存储每个电视节目的开始时间和结束时间。`cmp` 函数是用来比较两个电视节目的结束时间的,用于 `qsort` 排序。在 `main` 函数中,我们使用 `scanf` 读入每个测试用例,然后按照结束时间从早到晚对所有电视节目进行排序。接下来,我们使用 `count` 记录可以观看的电视节目数量,`end` 记录上一个观看的电视节目的结束时间。然后遍历所有电视节目,如果当前电视节目的开始时间大于等于 `end`,则选择该电视节目,并更新 `count` 和 `end`。最后输出 `count` 即可。
希望我的回答对您有帮助!
相关推荐
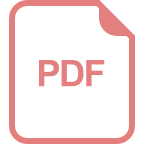
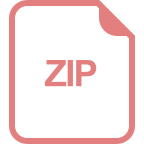
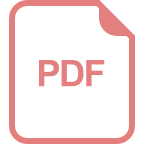












