分析下面代码动态规划的算法思路和相关逻辑 #include <iostream> #include <cstring> #include <algorithm> using namespace std; int profit[4][7] = { {0, 0, 0, 0, 0, 0, 0}, {0, 3, 5, 6, 7, 6, 5}, {0, 4, 6, 7, 8, 9, 10}, {0, 2, 5, 9, 8, 8, 7}}; int dp[10][10][10]; int main() { memset(dp, 0, sizeof(dp)); for (int i = 0; i < 7; i++) for (int j = 0; j < 7; j++) for (int k = 0; k < 7; k++) { dp[i][j][k] = max(dp[i][j][k], profit[1][i] + profit[2][j] + profit[3][k]); } int l = -1, r = -1, m = -1, res = -1; for (int i = 0; i < 7; i++) for (int j = 0; j < 7; j++) for (int k = 0; k < 7; k++) if ((i + k + j <= 6 && i + k + j >= 4) && res < dp[i][j][k]) { res = dp[i][j][k]; l = i; r = j; m = k; } printf("%d\n", l + r + m); printf("%d %d %d\n", l, r, m); printf("%d", res); return 0; }
时间: 2024-04-28 07:23:59 浏览: 47
这段代码是一个三元组问题的动态规划算法实现。该问题描述如下:有三个长度为7的数组A、B、C,每个数组分别包含7个数字。现在需要从三个数组中各选一个数字,组成一个三元组。要求选出的三元组中,所有数字的下标之和在4到6之间,且三元组中数字的和最大。该算法的思路如下:
1. 首先定义一个profit数组,用来存储三个数组中的数字。其中,profit[i][j]表示第i个数组中的第j个数字。
2. 定义一个dp数组,用来存储所有可能的三元组之和。其中,dp[i][j][k]表示从A数组中选出第i个数字,从B数组中选出第j个数字,从C数组中选出第k个数字时的三元组之和。
3. 遍历所有可能的三元组,并将它们的和存储到dp数组中。
4. 遍历dp数组,找到所有满足下标之和在4到6之间的三元组,并记录其中和最大的三元组和对应的下标。
5. 输出结果。
该算法的时间复杂度为O(n^3),其中n为数组长度。在本例中,n为7,因此该算法的时间复杂度为O(7^3)=O(343)。该算法的空间复杂度为O(n^3),由于本例中n为7,因此空间复杂度为O(7^3)=O(343)。
相关问题
#include <iostream> #include <fstream> #include <cstdio> #include <cstdlib> #include <cstring> #include <cmath> #include <climits> #include <string> #include <algorithm> #include <vector> #include <map> using namespace std; struct Value { char str[10000]; }; void structSort(Value *a, int n) { @你的代码 } int n; Value a[5000]; int main() { scanf("%d", &n); for (int i = 0; i<n; i++) { scanf("%s", a[i].str); } structSort(a, n); for (int i = 0; i<n; i++) { printf("%s\n", a[i].str); } return 0; }
这段代码给出了一个结构体数组 `a`,其中每个结构体包含一个字符串成员 `str`。接下来有一个函数 `structSort`,它需要你来实现。你需要使用任何一种排序算法对 `a` 数组进行排序,排序的依据是字符串的字典序(即按照字母顺序进行比较)。最后将排序后的字符串数组依次输出即可。
以下是可能的 `structSort` 实现方式之一,使用了标准库中的 `qsort` 函数:
```c++
int compare(const void *a, const void *b) {
return strcmp(((Value *)a)->str, ((Value *)b)->str);
}
void structSort(Value *a, int n) {
qsort(a, n, sizeof(Value), compare);
}
```
其中,`compare` 函数用于比较两个字符串的大小,将其作为参数传递给 `qsort` 函数进行排序。
#include<iostream> #include<algorithm> #include<vector> #include<deque> #include<map> #include<cstring> using namespace std; int main() { vector<int> v(10); int num; vector<int>::iterator beg = v.begin(); vector<int>::iterator end = v.end(); vector<int>::iterator mid = v.begin() + (end - beg) / 2; for (int i = 0; i < 10; i++) { v[i] = i; } cin >> num; sort(v.begin(), v.end()); while (*mid != num && beg <= end) { if (num < *mid) { end = mid; } else { beg = mid + 1; } mid = beg + (end - beg) / 2; } if (*mid == num) { cout << "Find" << endl; } else { cout << "Not Find" << endl; } return 0; }翻译每一行代码的意思
这段代码是一个使用二分查找算法在已排序的向量中查找指定值的示例程序。下面是每一行代码的意思:
1. `#include<iostream>`:包含输入输出流库。
2. `#include<algorithm>`:包含算法库。
3. `#include<vector>`:包含向量库。
4. `#include<deque>`:包含双端队列库。
5. `#include<map>`:包含映射库。
6. `#include<cstring>`:包含字符串库。
7. `using namespace std;`:使用标准命名空间。
8. `int main()`:主函数入口。
9. `vector<int> v(10);`:定义一个具有10个元素的整型向量v。
10. `int num;`:定义一个整型变量num。
11. `vector<int>::iterator beg = v.begin();`:定义一个向量迭代器beg,指向向量v的起始位置。
12. `vector<int>::iterator end = v.end();`:定义一个向量迭代器end,指向向量v的结束位置。
13. `vector<int>::iterator mid = v.begin() + (end - beg) / 2;`:定义一个向量迭代器mid,指向向量v的中间位置。
14. `for (int i = 0; i < 10; i++)`:循环10次,将向量v的元素赋值为索引值。
15. `v[i] = i;`:将向量v的第i个元素赋值为i。
16. `cin >> num;`:从标准输入读取一个整数并赋值给变量num。
17. `sort(v.begin(), v.end());`:对向量v进行排序。
18. `while (*mid != num && beg <= end)`:循环条件,mid指向的元素不等于num且beg小于等于end。
19. `if (num < *mid)`:如果num小于mid指向的元素。
20. `end = mid;`:将end更新为mid。
21. `else`:否则。
22. `beg = mid + 1;`:将beg更新为mid+1。
23. `mid = beg + (end - beg) / 2;`:将mid更新为beg与end的中间位置。
24. `if (*mid == num)`:如果mid指向的元素等于num。
25. `cout << "Find" << endl;`:输出"Find"。
26. `else`:否则。
27. `cout << "Not Find" << endl;`:输出"Not Find"。
28. `return 0;`:返回0,表示程序正常结束。
相关推荐
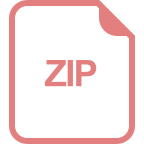













