JAVA求给定矩阵的全部局部极大值及其所在的位置。
时间: 2024-05-08 20:17:47 浏览: 80
以下是一个示例代码,用于查找给定矩阵的全部局部极大值及其所在的位置。
```
public class LocalMaxima {
public static void main(String[] args) {
int[][] matrix = {
{1, 2, 3, 4},
{5, 6, 7, 8},
{9, 10, 11, 12},
{13, 14, 15, 16}
};
List<Point> localMaximas = findLocalMaximas(matrix);
for (Point p : localMaximas) {
System.out.println("Local maximum value: " + matrix[p.x][p.y] + ", at position: (" + p.x + "," + p.y + ")");
}
}
public static List<Point> findLocalMaximas(int[][] matrix) {
List<Point> localMaximas = new ArrayList<Point>();
int rows = matrix.length;
int cols = matrix[0].length;
for (int i = 1; i < rows - 1; i++) {
for (int j = 1; j < cols - 1; j++) {
int currentValue = matrix[i][j];
if (currentValue > matrix[i - 1][j] &&
currentValue > matrix[i + 1][j] &&
currentValue > matrix[i][j - 1] &&
currentValue > matrix[i][j + 1]) {
localMaximas.add(new Point(i, j));
}
}
}
return localMaximas;
}
}
```
该示例代码中,我们首先定义了一个二维矩阵,并且调用了 `findLocalMaximas` 方法来查找这个矩阵的全部局部极大值。`findLocalMaximas` 方法遍历了矩阵的每一个元素,并且判断了该元素是否为局部极大值。在这个方法中,我们通过比较当前元素与其相邻元素的大小关系来判断它是否为局部极大值。如果当前元素大于其相邻元素,则该元素为局部极大值。最后,我们将全部局部极大值的位置保存在一个 `List` 中,并将其返回。
在上述示例中,我们使用了 `Point` 类来表示一个坐标点。如果你不熟悉 `Point` 类,可以使用 `int[]` 或 `List<Integer>` 等数据结构来保存坐标点。
阅读全文
相关推荐
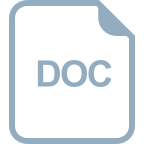
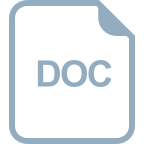
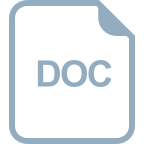



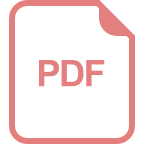
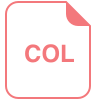
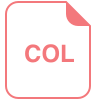
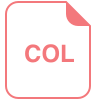
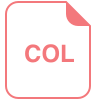
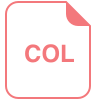
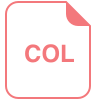
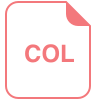
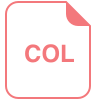
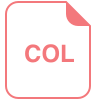
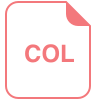
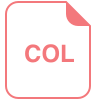
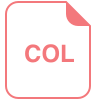