人工免疫算法求解TSP,写一段python代码
时间: 2023-10-24 07:30:33 浏览: 49
好的,以下是一个简单的人工免疫算法求解TSP的Python代码实现:
```python
import random
import math
# 定义TSP问题的距离矩阵
distance_matrix = [
[0, 2, 9, 10],
[1, 0, 6, 4],
[15, 7, 0, 8],
[6, 3, 12, 0]
]
# 定义参数
N = 10 # 种群规模
G = 100 # 迭代次数
beta = 2 # 信度水平
p = 0.1 # 免疫率
d = 0.9 # 丧失率
# 初始化种群
population = []
for i in range(N):
chromosome = list(range(len(distance_matrix)))
random.shuffle(chromosome)
population.append(chromosome)
# 计算每个个体的适应度值
def evaluate(chromosome):
distance = 0
for i in range(len(chromosome)-1):
distance += distance_matrix[chromosome[i]][chromosome[i+1]]
distance += distance_matrix[chromosome[-1]][chromosome[0]]
return 1 / distance
# 进行GA操作,包括选择、交叉、变异
def genetic_algorithm(population):
# 选择
population = sorted(population, key=lambda x: evaluate(x), reverse=True)
elite = population[0]
parents = population[:int(N/2)]
# 交叉
children = []
for i in range(int(N/2)):
parent1 = random.choice(parents)
parent2 = random.choice(parents)
child1, child2 = order_crossover(parent1, parent2)
children += [child1, child2]
# 变异
for i in range(N):
if random.random() < p:
children[i] = inversion_mutation(children[i])
# 合并父代、子代,选择前N个
population = parents + children
population = sorted(population, key=lambda x: evaluate(x), reverse=True)
return population[:N]
# 顺序交叉
def order_crossover(parent1, parent2):
child1, child2 = [-1]*len(parent1), [-1]*len(parent2)
left, right = sorted([random.randrange(len(parent1)) for _ in range(2)])
for i in range(left, right+1):
child1[i] = parent1[i]
child2[i] = parent2[i]
idx1, idx2 = right+1, right+1
while -1 in child1:
if parent2[idx1%len(parent2)] not in child1:
child1[idx1%len(parent2)] = parent2[idx1%len(parent2)]
idx1 += 1
while -1 in child2:
if parent1[idx2%len(parent1)] not in child2:
child2[idx2%len(parent1)] = parent1[idx2%len(parent1)]
idx2 += 1
return child1, child2
# 反转变异
def inversion_mutation(chromosome):
left, right = sorted([random.randrange(len(chromosome)) for _ in range(2)])
return chromosome[:left] + chromosome[left:right+1][::-1] + chromosome[right+1:]
# 初始化抗体群
antibodies = []
for i in range(N):
chromosome = list(range(len(distance_matrix)))
random.shuffle(chromosome)
antibodies.append(chromosome)
# 进行人工免疫算法操作
for g in range(G):
# 计算每个抗体的亲和度
affinities = [evaluate(antibody) for antibody in antibodies]
# 选择免疫记忆库中的抗体
selected_antibodies = []
for i in range(N):
if random.random() < d:
selected_antibodies.append(population[i])
else:
selected_antibodies.append(antibodies[i])
# 交叉变异
for i in range(N):
parent1 = random.choice(selected_antibodies)
parent2 = random.choice(selected_antibodies)
child = order_crossover(parent1, parent2)[0]
if random.random() < p:
child = inversion_mutation(child)
antibodies[i] = child
# 更新免疫记忆库
population = genetic_algorithm(population + antibodies)
# 输出最优解
print("最优解:", population[0])
print("最优解的距离:", 1/evaluate(population[0]))
```
这段代码实现了一个简单的人工免疫算法,其中包括了遗传算法操作和抗体操作,用于求解TSP问题。在代码中,我们首先定义了TSP问题的距离矩阵,然后初始化了种群和抗体群。在每次迭代中,我们计算了每个抗体的适应度值,并选择了免疫记忆库中的抗体进行交叉变异操作。最后,我们用遗传算法对免疫记忆库进行更新,并输出最优解。
相关推荐
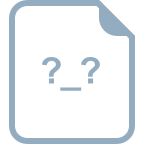
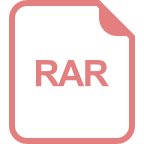














