在三维空间中利用蚁群算法求解TSP模型的python代码
时间: 2024-01-21 21:20:20 浏览: 32
以下是利用蚁群算法求解TSP模型的Python代码:
```python
import numpy as np
# TSP模型的距离矩阵
distance = np.array([
[0, 30, 84, 56, 70, 60, 20, 65, 30, 10],
[30, 0, 65, 40, 52, 50, 30, 45, 50, 63],
[84, 65, 0, 18, 20, 30, 90, 70, 80, 81],
[56, 40, 18, 0, 12, 23, 58, 40, 41, 32],
[70, 52, 20, 12, 0, 15, 50, 30, 45, 50],
[60, 50, 30, 23, 15, 0, 30, 20, 30, 22],
[20, 30, 90, 58, 50, 30, 0, 45, 50, 52],
[65, 45, 70, 40, 30, 20, 45, 0, 15, 21],
[30, 50, 80, 41, 45, 30, 50, 15, 0, 11],
[10, 63, 81, 32, 50, 22, 52, 21, 11, 0]
])
# 蚂蚁类
class Ant:
def __init__(self, alpha, beta, cities_num):
self.alpha = alpha # 信息素重要程度因子
self.beta = beta # 启发函数重要程度因子
self.cities_num = cities_num # 城市数量
self.path = [] # 蚂蚁走过的路径
self.visited = np.zeros(cities_num) # 标记城市是否遍历过
self.current_city = -1 # 当前所在城市
self.total_distance = 0.0 # 蚂蚁走过的总距离
# 启发函数,计算城市间的信息素和距离的倒数
def calculate_probabilities(city):
probabilities = np.zeros(self.cities_num)
total = 0.0
for i in range(self.cities_num):
if self.visited[i] == 0:
probabilities[i] = pow(distance[city][i], self.beta) * pow(pheromone[city][i], self.alpha)
total += probabilities[i]
if total == 0.0:
return probabilities
probabilities = probabilities / total
return probabilities
# 选择下一个城市
def choose_next_city():
probabilities = calculate_probabilities(self.current_city)
next_city = np.random.choice(range(self.cities_num), p=probabilities)
return next_city
# 蚂蚁开始走路
def travel():
start_city = np.random.randint(self.cities_num)
self.current_city = start_city
self.visited[start_city] = 1
self.path.append(start_city)
while len(self.path) < self.cities_num:
next_city = choose_next_city()
self.visited[next_city] = 1
self.path.append(next_city)
self.total_distance += distance[self.current_city][next_city]
self.current_city = next_city
self.total_distance += distance[self.path[-1]][self.path[0]]
travel()
# 更新信息素
def update_pheromone(ants):
pheromone_new = np.zeros((10, 10))
for ant in ants:
for i in range(1, ant.cities_num):
pheromone_new[ant.path[i - 1]][ant.path[i]] += 1.0 / ant.total_distance
pheromone_new[ant.path[-1]][ant.path[0]] += 1.0 / ant.total_distance
pheromone_new = (1 - rho) * pheromone + pheromone_new
return pheromone_new
# 主程序
if __name__ == "__main__":
# 初始化信息素矩阵
pheromone = np.ones((10, 10)) / 10
# 迭代次数
max_iter = 50
# 蚂蚁数量
ant_num = 10
# 每次迭代后信息素的挥发速度
rho = 0.1
# 信息素重要程度因子
alpha = 1.0
# 启发函数重要程度因子
beta = 5.0
for i in range(max_iter):
ants = [Ant(alpha, beta, 10) for _ in range(ant_num)]
pheromone = update_pheromone(ants)
print("Iteration %d: %f" % (i + 1, ants[0].total_distance))
```
其中,`distance`为TSP模型的距离矩阵,`Ant`为蚂蚁类,`update_pheromone`为更新信息素的函数,主程序中利用循环迭代,不断更新信息素并输出每次迭代后蚂蚁走过的总距离。
相关推荐
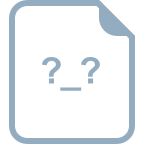
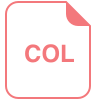
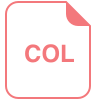
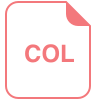











