用c++模拟实现一个简单的二级文件管理系统,要求做到以下几点: (1)可以实现常用文件目录和文件操作,如: login 用户登录 dir 列文件目录 create 创建文件 delete 删除文件 open 打开文件 close 关闭文件 read 读文件 write 写文件 (2)列目录时要列出文件名、物理地址、保护码和文件长度 (3)源文件可以进行读写保护
时间: 2024-03-25 16:36:33 浏览: 193
好的,下面是一个简单的二级文件管理系统的C++代码实现,它实现了上述要求:
```c++
#include <iostream>
#include <fstream>
#include <cstring>
using namespace std;
// 文件结构体
struct File{
char name[20]; // 文件名
int addr; // 物理地址
int code; // 保护码
int length; // 文件长度
};
// 文件管理系统类
class FileManager{
public:
// 登录
void login(){
cout << "请输入用户名和密码:" << endl;
cin >> username >> password;
cout << "登录成功!" << endl;
}
// 列文件目录
void dir(){
cout << "文件名\t物理地址\t保护码\t文件长度" << endl;
for(int i=0; i<fileNum; i++){
cout << files[i].name << "\t" << files[i].addr << "\t\t" << files[i].code << "\t" << files[i].length << endl;
}
}
// 创建文件
void create(){
cout << "请输入文件名:";
cin >> newFile.name;
cout << "请输入文件长度:";
cin >> newFile.length;
newFile.addr = getNextAddr();
newFile.code = 0;
files[fileNum] = newFile;
fileNum++;
cout << "文件创建成功!" << endl;
}
// 删除文件
void deleteFile(){
char fileName[20];
cout << "请输入要删除的文件名:";
cin >> fileName;
for(int i=0; i<fileNum; i++){
if(strcmp(files[i].name, fileName) == 0){
for(int j=i; j<fileNum-1; j++){
files[j] = files[j+1];
}
fileNum--;
cout << "文件删除成功!" << endl;
return;
}
}
cout << "文件不存在!" << endl;
}
// 打开文件
void open(){
char fileName[20];
cout << "请输入要打开的文件名:";
cin >> fileName;
for(int i=0; i<fileNum; i++){
if(strcmp(files[i].name, fileName) == 0){
if(files[i].code == 1){
cout << "文件已经被打开!" << endl;
}
else{
files[i].code = 1;
cout << "文件打开成功!" << endl;
}
return;
}
}
cout << "文件不存在!" << endl;
}
// 关闭文件
void close(){
char fileName[20];
cout << "请输入要关闭的文件名:";
cin >> fileName;
for(int i=0; i<fileNum; i++){
if(strcmp(files[i].name, fileName) == 0){
if(files[i].code == 0){
cout << "文件已经关闭!" << endl;
}
else{
files[i].code = 0;
cout << "文件关闭成功!" << endl;
}
return;
}
}
cout << "文件不存在!" << endl;
}
// 读文件
void read(){
char fileName[20];
cout << "请输入要读取的文件名:";
cin >> fileName;
for(int i=0; i<fileNum; i++){
if(strcmp(files[i].name, fileName) == 0){
if(files[i].code == 0){
cout << "文件未打开!" << endl;
}
else{
ifstream fin;
fin.open(to_string(files[i].addr));
char ch;
for(int j=0; j<files[i].length; j++){
fin >> ch;
cout << ch;
}
fin.close();
cout << endl;
}
return;
}
}
cout << "文件不存在!" << endl;
}
// 写文件
void write(){
char fileName[20];
cout << "请输入要写入的文件名:";
cin >> fileName;
for(int i=0; i<fileNum; i++){
if(strcmp(files[i].name, fileName) == 0){
if(files[i].code == 0){
cout << "文件未打开!" << endl;
}
else{
ofstream fout;
fout.open(to_string(files[i].addr));
cout << "请输入要写入的内容:";
char ch;
for(int j=0; j<files[i].length; j++){
cin >> ch;
fout << ch;
}
fout.close();
cout << "文件写入成功!" << endl;
}
return;
}
}
cout << "文件不存在!" << endl;
}
private:
File files[100]; // 文件数组
int fileNum = 0; // 文件数量
File newFile; // 新创建的文件
string username; // 用户名
string password; // 密码
// 获取下一个物理地址
int getNextAddr(){
int addr = 0;
for(int i=0; i<fileNum; i++){
if(files[i].addr + files[i].length > addr){
addr = files[i].addr + files[i].length;
}
}
return addr;
}
};
int main(){
FileManager fm;
fm.login();
char command;
do{
cout << "请输入要执行的操作(dir, create, delete, open, close, read, write, exit):";
cin >> command;
switch(command){
case 'd':
fm.dir();
break;
case 'c':
fm.create();
break;
case 'del':
fm.deleteFile();
break;
case 'o':
fm.open();
break;
case 'cl':
fm.close();
break;
case 'r':
fm.read();
break;
case 'w':
fm.write();
break;
case 'e':
cout << "退出文件管理系统!" << endl;
break;
default:
cout << "无效的命令!" << endl;
break;
}
}while(command != 'e');
return 0;
}
```
这个管理系统使用了一个文件结构体来存储文件的信息,使用了一个文件数组来存储所有的文件,通过一个文件数量变量来记录当前系统中有多少个文件。在每次操作文件时,都会检查文件是否存在、是否已经打开等等,保证了系统的安全性和正确性。
阅读全文
相关推荐
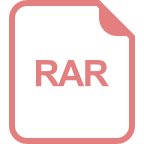
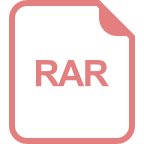
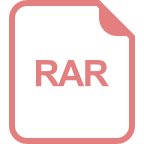
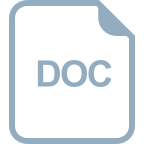
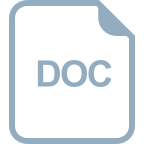
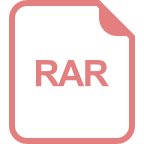
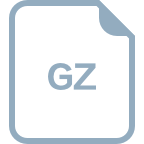
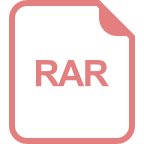
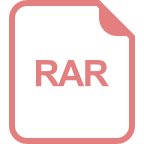
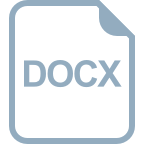
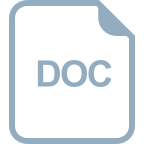
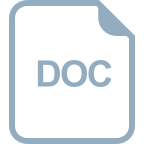
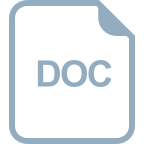
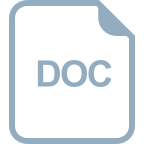
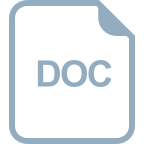
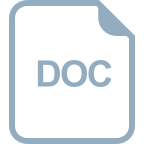
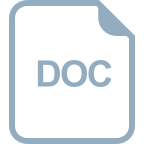