javaswing转盘指针抽奖
时间: 2023-06-22 18:18:14 浏览: 51
您可以按照以下步骤使用Java Swing创建一个简单的转盘抽奖程序:
1. 创建一个JFrame,并将其设置为GridLayout,以便在其中放置转盘和指针。
2. 在JFrame中创建一个JPanel,用于绘制转盘。您可以使用Graphics类的drawArc方法来绘制圆形部分,并使用drawLine方法绘制指针。您还可以使用FontMetrics类来计算文本的宽度和高度,以便在转盘上显示文本。
3. 创建一个ActionListener,用于处理抽奖按钮的点击事件。当用户单击按钮时,该监听器应该计算随机数,并将指针旋转到相应的位置。
4. 将转盘和抽奖按钮添加到JFrame中,并显示该窗口。
以下是一个简单的示例代码,可以帮助您开始编写您的转盘抽奖程序:
```java
import java.awt.Color;
import java.awt.Font;
import java.awt.FontMetrics;
import java.awt.Graphics;
import java.awt.GridLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JPanel;
public class LuckyDraw extends JFrame {
private static final long serialVersionUID = 1L;
private static final int PANEL_WIDTH = 500;
private static final int PANEL_HEIGHT = 500;
private static final int NUM_SECTORS = 8;
private static final int ANGLE_INCREMENT = 360 / NUM_SECTORS;
private static final int POINTER_LENGTH = 200;
private JPanel panel;
private JButton button;
public LuckyDraw() {
setTitle("Lucky Draw");
setSize(PANEL_WIDTH, PANEL_HEIGHT);
setDefaultCloseOperation(EXIT_ON_CLOSE);
setLocationRelativeTo(null);
setLayout(new GridLayout(2, 1));
panel = new JPanel() {
private static final long serialVersionUID = 1L;
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
int x = getWidth() / 2;
int y = getHeight() / 2;
int radius = Math.min(getWidth(), getHeight()) / 2 - 50;
g.setColor(Color.WHITE);
g.fillArc(x - radius, y - radius, radius * 2, radius * 2, 0, 360);
g.setColor(Color.BLACK);
g.drawArc(x - radius, y - radius, radius * 2, radius * 2, 0, 360);
for (int i = 0; i < NUM_SECTORS; i++) {
int startAngle = i * ANGLE_INCREMENT;
int endAngle = (i + 1) * ANGLE_INCREMENT;
g.setColor(getSectorColor(i));
g.fillArc(x - radius, y - radius, radius * 2, radius * 2, startAngle, ANGLE_INCREMENT);
g.setColor(Color.BLACK);
g.drawArc(x - radius, y - radius, radius * 2, radius * 2, startAngle, ANGLE_INCREMENT);
drawSectorText(g, i, startAngle, endAngle, x, y, radius);
}
g.setColor(Color.RED);
g.drawLine(x, y, (int) (x + POINTER_LENGTH * Math.cos(Math.toRadians(0))),
(int) (y - POINTER_LENGTH * Math.sin(Math.toRadians(0))));
}
};
button = new JButton("Lucky Draw");
button.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
int sector = (int) (Math.random() * NUM_SECTORS);
int angle = sector * ANGLE_INCREMENT + (int) (Math.random() * ANGLE_INCREMENT);
panel.getGraphics().setColor(Color.RED);
panel.getGraphics().drawLine(PANEL_WIDTH / 2, PANEL_HEIGHT / 2,
(int) (PANEL_WIDTH / 2 + POINTER_LENGTH * Math.cos(Math.toRadians(angle))),
(int) (PANEL_HEIGHT / 2 - POINTER_LENGTH * Math.sin(Math.toRadians(angle))));
}
});
add(panel);
add(button);
setVisible(true);
}
private Color getSectorColor(int sector) {
switch (sector) {
case 0:
return Color.YELLOW;
case 1:
return Color.GREEN;
case 2:
return Color.ORANGE;
case 3:
return Color.BLUE;
case 4:
return Color.PINK;
case 5:
return Color.MAGENTA;
case 6:
return Color.CYAN;
case 7:
return Color.DARK_GRAY;
default:
return Color.WHITE;
}
}
private void drawSectorText(Graphics g, int sector, int startAngle, int endAngle, int x, int y, int radius) {
String text = "Sector " + sector;
Font font = new Font("Arial", Font.BOLD, 16);
g.setFont(font);
FontMetrics metrics = g.getFontMetrics(font);
int textWidth = metrics.stringWidth(text);
int textHeight = metrics.getHeight();
double angle = Math.toRadians((startAngle + endAngle) / 2.0);
int cx = (int) (x + (radius - textHeight) * Math.cos(angle));
int cy = (int) (y - (radius - textHeight) * Math.sin(angle));
g.drawString(text, cx - textWidth / 2, cy + textHeight / 2);
}
public static void main(String[] args) {
new LuckyDraw();
}
}
```
希望这可以帮助您开始编写Java Swing转盘抽奖程序。
相关推荐
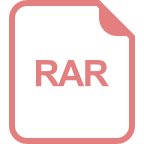














