python 网页下载文件,并重命名
时间: 2024-11-10 09:25:16 浏览: 14
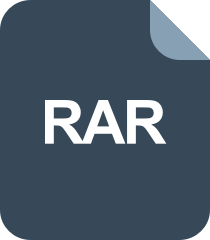
用Python批量重命名文件

在Python中,你可以使用`requests`库来下载网页内容,然后使用`os`库进行文件的重命名操作。下面是一个简单的示例:
```python
import requests
from os import path
def download_and_rename(url, target_filename):
# 下载文件
response = requests.get(url)
# 检查请求状态码,如果成功则继续,失败则返回错误
if response.status_code == 200:
# 获取原始文件名(如果有)
original_name = path.basename(url) if url.endswith('/') else path.splitext(url)[1]
# 设置目标文件路径,如果没有指定,则默认当前目录下
target_path = path.join(path.dirname(target_filename), original_name)
with open(target_path, 'wb') as f:
f.write(response.content)
# 重命名文件
new_filename = "new_file_name" + original_name # 可以自定义新的文件名格式
if not path.exists(new_filename): # 检查新名称是否已存在
shutil.move(target_path, new_filename)
print(f"File downloaded and renamed to {new_filename}")
else:
print("Error: New file name already exists.")
else:
print(f"Failed to download the file. Status code: {response.status_code}")
# 调用函数并传入URL和目标文件的新名称
download_and_rename('http://example.com/file.pdf', 'target_directory/new_file_name.pdf')
```
在这个例子中,`download_and_rename`函数接受一个URL和目标文件的新名称作为输入,下载对应URL的文件,将其保存到指定的目标文件夹,并按照新的名称重命名。
阅读全文
相关推荐
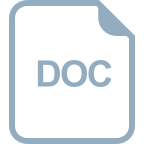
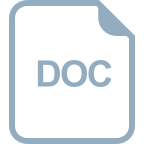
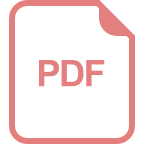














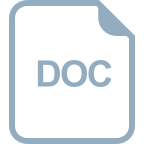